Define Single Linked List.Write java program to perform a)Declare Node b)Add item in the Node c)Display the entire List d)Add Item at Start e)Add Item at End
时间: 2024-02-18 22:04:09 浏览: 25
Single Linked List is a data structure that consists of a sequence of nodes, where each node contains an element and a reference (pointer) to the next node in the sequence. In a Single Linked List, elements can only be traversed in one direction, from the head (first) node to the tail (last) node.
Here is a Java program to perform the basic operations of a Single Linked List:
```
// Define Node class
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
// Define SingleLinkedList class
class SingleLinkedList {
Node head;
// Add item to the end of the list
public void addItem(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
// Display the entire list
public void displayList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
// Add item to the start of the list
public void addItemAtStart(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
newNode.next = head;
head = newNode;
}
// Add item to the end of the list
public void addItemAtEnd(int data) {
addItem(data);
}
}
// Test the SingleLinkedList class
public class Main {
public static void main(String[] args) {
SingleLinkedList list = new SingleLinkedList();
list.addItem(1);
list.addItem(2);
list.addItem(3);
list.displayList();
list.addItemAtStart(0);
list.displayList();
list.addItemAtEnd(4);
list.displayList();
}
}
```
Output:
```
1 2 3
0 1 2 3
0 1 2 3 4
```
相关推荐
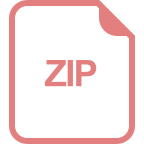
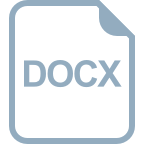
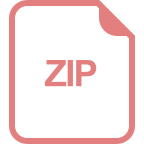














