please generate a C++ program that creates a tree node in a certain place of a tree.
时间: 2024-05-07 11:20:34 浏览: 115

树结构示例C++程序
Here is an example C++ program that creates a tree node at a specific position in a tree:
```c++
#include <iostream>
#include <vector>
using namespace std;
struct TreeNode {
int val;
vector<TreeNode*> children;
TreeNode(int x) : val(x) {}
};
void createNode(TreeNode* root, vector<int> path, int pos, int val) {
if (pos == path.size()) {
root->children.push_back(new TreeNode(val));
return;
}
int index = path[pos];
createNode(root->children[index], path, pos + 1, val);
}
int main() {
TreeNode* root = new TreeNode(1);
root->children.push_back(new TreeNode(2));
root->children.push_back(new TreeNode(3));
root->children.push_back(new TreeNode(4));
vector<int> path = {1, 0}; // path to the node we want to create
int val = 5; // value of the new node
createNode(root, path, 0, val);
// print the tree to verify the new node was added
cout << root->val << endl;
for (auto child : root->children) {
cout << " " << child->val << endl;
for (auto grandchild : child->children) {
cout << " " << grandchild->val << endl;
}
}
return 0;
}
```
In this program, we define a `TreeNode` struct that represents a node in a tree. Each node has a `val` property representing its value, and a `children` vector representing its children.
The `createNode` function takes a `root` parameter representing the root of the tree, a `path` parameter representing the path to the node we want to create (as a vector of indices), a `pos` parameter representing the current position in the path, and a `val` parameter representing the value of the new node. The function recursively traverses the tree along the path until it reaches the position where the new node should be created, and then adds the new node as a child of the current node.
In the `main` function, we create a sample tree and then call the `createNode` function to add a new node with value 5 at position [1, 0] (i.e., the second child of the root's first child). We then print the tree to verify that the new node was added correctly.
阅读全文
相关推荐
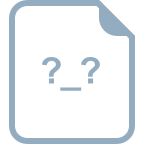
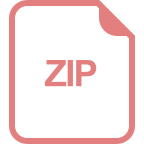
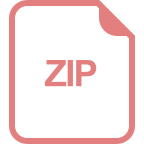
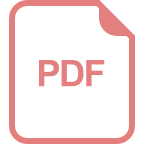
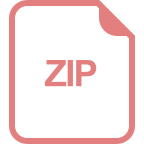
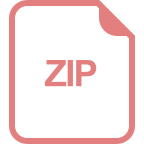
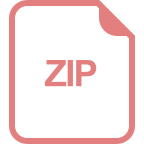
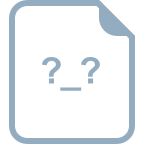
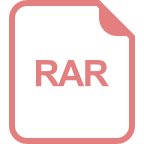
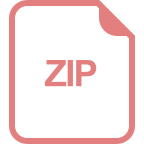
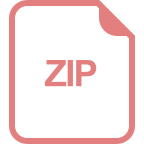
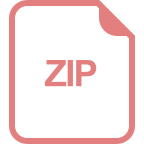
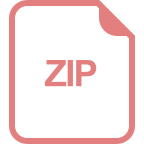