write a python code that can generate a Christmas tree
时间: 2023-09-03 17:25:58 浏览: 74
### 回答1:
Sure! Here's an example Python code to generate a Christmas tree using the `print()` function:
```python
# Define the height of the tree
height = 10
# Print the top of the tree
print(' '*(height-1) + '*')
# Print the middle of the tree
for i in range(2, height+1):
print(' '*(height-i) + '*'*(2*i-1))
# Print the base of the tree
print(' '*(height-2) + '***')
```
You can adjust the value of `height` to generate a tree of different heights. The output will look like this for `height=10`:
```
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
***
```
### 回答2:
以下是一个使用Python编写的生成圣诞树的代码:
```python
def generate_christmas_tree(height):
for i in range(height):
print(' ' * (height - i - 1) + '*' * (2 * i + 1))
print(' ' * (height - 1) + '|')
height = int(input("请输入圣诞树的高度:"))
generate_christmas_tree(height)
```
该代码使用了一个for循环来生成每一行的圣诞树形状,并使用print语句在控制台上打印出来。
在函数generate_christmas_tree中,我们使用内层循环打印出适当数量的空格和星号,以形成每一行的形状。外层循环根据树的高度重复生成每个行。最后一行是树干,它是由一个空格和一个竖线组成的。
在主程序中,我们使用input函数来获取用户输入的树的高度,并将其作为参数传递给generate_christmas_tree函数。
### 回答3:
下面是一个可以生成圣诞树的Python代码:
```python
def generate_tree(height):
for i in range(height):
print(' ' * (height - i - 1) + '*' * (2 * i + 1))
print(' ' * (height - 1) + '|')
height = int(input('请输入圣诞树的高度:'))
generate_tree(height)
```
这个代码使用了一个循环来生成每一层的圣诞树形状,并通过空格和星号字符来形成树的形状。最后一行用竖线字符作为树干。您可以输入一个整数作为高度,代码将根据所输入的高度生成相应高度的圣诞树。
相关推荐
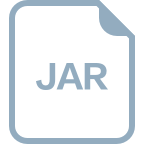
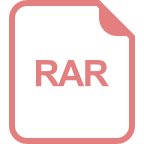
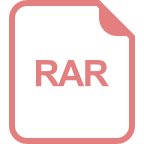














