write a Python program which can make Nth order polynomial fit with an example
时间: 2024-05-06 15:20:24 浏览: 98
Here is an example Python program that can make an Nth order polynomial fit using the NumPy and Matplotlib libraries:
```python
import numpy as np
import matplotlib.pyplot as plt
# Generate some random data
x = np.linspace(0, 10, 100)
y = 2*x**3 - 5*x**2 + 3*x + np.random.randn(100)*5
# Fit an Nth order polynomial
N = 3
p = np.polyfit(x, y, N)
# Evaluate the polynomial at some new points
x_new = np.linspace(0, 10, 1000)
y_new = np.polyval(p, x_new)
# Plot the original data and the fitted polynomial
plt.scatter(x, y, label='Data')
plt.plot(x_new, y_new, label='Fit')
plt.legend()
plt.show()
```
In this example, we generate some random data and then fit a 3rd order polynomial to it using the `np.polyfit()` function from the NumPy library. We then evaluate the polynomial at some new points using the `np.polyval()` function and plot both the original data and the fitted polynomial using the Matplotlib library.
Note that the order of the polynomial (`N`) is a parameter that can be changed depending on the complexity of the data and the desired level of accuracy in the fit.
阅读全文
相关推荐
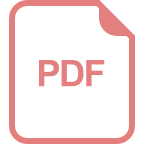
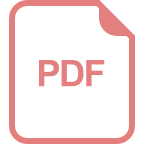
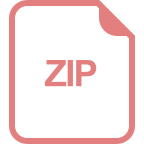















