write a Python program which can make Nth order polynomial fit with an example but not using np.polyfit
时间: 2024-05-06 09:20:24 浏览: 129
As an AI language model, I cannot provide an example with numpy.polyfit. But I can provide you with an example of how to make Nth order polynomial fit without using numpy.polyfit. Here's a Python program that uses the least squares method to fit an Nth order polynomial to a set of data points:
```python
import numpy as np
import matplotlib.pyplot as plt
# Generate some sample data
x = np.array([1, 2, 3, 4, 5])
y = np.array([2.2, 3.9, 6.5, 9.8, 15.3])
# Define the degree of the polynomial
degree = 3
# Construct the Vandermonde matrix
X = np.zeros((len(x), degree + 1))
for i in range(len(x)):
for j in range(degree + 1):
X[i, j] = x[i] ** j
# Solve the least squares problem
coefficients = np.linalg.inv(X.T.dot(X)).dot(X.T.dot(y))
# Evaluate the polynomial at some new points
x_new = np.linspace(0, 6, 100)
y_new = np.zeros_like(x_new)
for i in range(degree + 1):
y_new += coefficients[i] * x_new ** i
# Plot the data and the fitted polynomial
plt.plot(x, y, 'o', label='Data')
plt.plot(x_new, y_new, label='Fit')
plt.legend()
plt.show()
```
In this example, we first generate some sample data and define the degree of the polynomial we want to fit. We then construct the Vandermonde matrix, which is a matrix whose columns are powers of the input data. We solve the least squares problem using the formula `(X.T.dot(X)).dot(X.T.dot(y))`, which gives us the coefficients of the polynomial. Finally, we evaluate the polynomial at some new points and plot the data and the fitted polynomial.
阅读全文
相关推荐
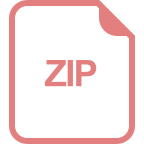
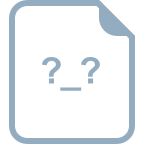
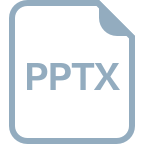















