用c语言编写一个算法计算返回链栈中结点的个数,每行单独分开并标有注释。
时间: 2024-10-22 21:05:11 浏览: 13
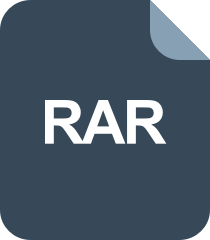
大学C语言实验经典编程题实现(包含详细算法思想描述和每一行代码注释)
在 C 语言中,我们可以使用栈数据结构来计算链表节点的数量。由于链表本身并不具备自动记录元素个数的功能,我们需要遍历整个链表并在遍历过程中计数。这里是一个简单的示例,包括函数定义、主函数和注释:
```c
#include <stdio.h> // 引入标准输入输出库,用于打印结果
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的链表节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
if (newNode != NULL) { // 如果分配成功
newNode->data = value;
newNode->next = NULL; // 初始化下一个节点为空
} else {
printf("Memory allocation failed!\n"); // 错误处理
return NULL;
}
return newNode;
}
// 计算链表节点总数的函数
int countNodes(Node* head) {
int count = 0; // 初始化节点计数为0
while (head != NULL) { // 当链表非空时,继续遍历
count++; // 每次遇到一个节点,计数加一
head = head->next; // 移动到下一个节点
}
return count; // 返回节点总数
}
// 主函数演示如何使用上面的函数
int main() {
Node* listHead = createNode(1); // 创建链表的第一个节点,值为1
listHead->next = createNode(2); // 添加第二个节点,值为2
listHead->next->next = createNode(3); // 添加第三个节点,值为3
int nodeCount = countNodes(listHead);
printf("The number of nodes in the linked list is: %d\n", nodeCount);
free(listHead); // 清理内存,防止内存泄漏
return 0;
}
```
在这个示例中,`countNodes` 函数通过遍历链表的每个节点,递增计数器 `count` 来得到节点总数。在 `main` 函数里,我们创建了一个包含三个节点的简单链表,并调用 `countNodes` 获取节点数。
阅读全文
相关推荐
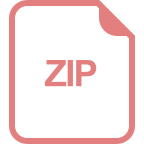
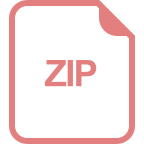
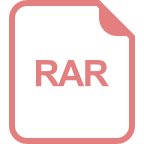
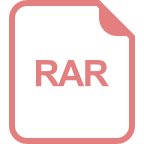
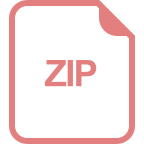
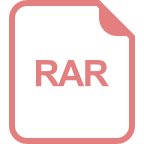
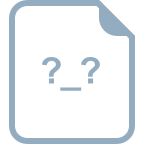
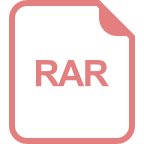
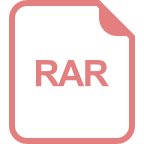
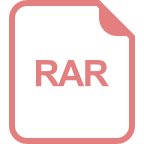
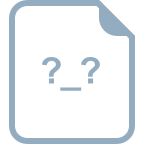