强化学习 自动驾驶 carla
时间: 2023-11-28 12:46:03 浏览: 246
强化学习是一种机器学习方法,它通过试错来学习如何在特定环境中采取行动以最大化奖励。CARLA是一个开源的自动驾驶仿真平台,可以用于测试和评估自动驾驶算法。下面是使用强化学习在CARLA中实现自动驾驶的一些步骤:
1. 安装CARLA和Python API
```shell
# 安装CARLA
wget https://carla-releases.s3.eu-west-3.amazonaws.com/Linux/CARLA_0.9.11.tar.gz
tar -xvf CARLA_0.9.11.tar.gz
# 安装Python API
pip install pygame numpy networkx scipy matplotlib
git clone https://github.com/carla-simulator/carla.git
cd carla/PythonAPI/carla/dist
easy_install carla-0.9.11-py3.7-linux-x86_64.egg
```
2. 创建CARLA环境
```python
import carla
# 连接到CARLA服务器
client = carla.Client('localhost', 2000)
client.set_timeout(10.0)
# 获取CARLA世界
world = client.get_world()
# 设置天气和时间
weather = carla.WeatherParameters(cloudiness=10.0, precipitation=10.0, sun_altitude_angle=70.0)
world.set_weather(weather)
world.set_sun_position(carla.Location(x=0.0, y=0.0, z=0.0))
# 创建车辆和摄像头
blueprint_library = world.get_blueprint_library()
vehicle_bp = blueprint_library.filter('vehicle.tesla.model3')[0]
spawn_point = carla.Transform(carla.Location(x=50.0, y=0.0, z=2.0), carla.Rotation(yaw=180.0))
vehicle = world.spawn_actor(vehicle_bp, spawn_point)
camera_bp = blueprint_library.find('sensor.camera.rgb')
camera_transform = carla.Transform(carla.Location(x=1.5, z=2.4))
camera = world.spawn_actor(camera_bp, camera_transform, attach_to=vehicle)
```
3. 实现强化学习算法
这里我们以Deep Q-Network (DQN)为例,使用Keras实现神经网络。
```python
import keras
from keras.models import Sequential
from keras.layers import Dense, Flatten
from keras.optimizers import Adam
class DQNAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.memory = deque(maxlen=2000)
self.gamma = 0.95
self.epsilon = 1.0
self.epsilon_min = 0.01
self.epsilon_decay = 0.995
self.learning_rate = 0.001
self.model = self._build_model()
def _build_model(self):
model = Sequential()
model.add(Flatten(input_shape=(1,) + self.state_size))
model.add(Dense(24, activation='relu'))
model.add(Dense(24, activation='relu'))
model.add(Dense(self.action_size, activation='linear'))
model.compile(loss='mse', optimizer=Adam(lr=self.learning_rate))
return model
def remember(self, state, action, reward, next_state, done):
self.memory.append((state, action, reward, next_state, done))
def act(self, state):
if np.random.rand() <= self.epsilon:
return random.randrange(self.action_size)
act_values = self.model.predict(state)
return np.argmax(act_values[0])
def replay(self, batch_size):
minibatch = random.sample(self.memory, batch_size)
for state, action, reward, next_state, done in minibatch:
target = reward
if not done:
target = (reward + self.gamma * np.amax(self.model.predict(next_state)[0]))
target_f = self.model.predict(state)
target_f[0][action] = target
self.model.fit(state, target_f, epochs=1, verbose=0)
if self.epsilon > self.epsilon_min:
self.epsilon *= self.epsilon_decay
# 初始化DQNAgent
state_size = (800, 600, 3)
action_size = 3
agent = DQNAgent(state_size, action_size)
# 训练DQNAgent
for e in range(EPISODES):
state = env.reset()
state = np.reshape(state, [1, 1] + list(state_size))
for time in range(500):
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, 1] + list(state_size))
agent.remember(state, action, reward, next_state, done)
state = next_state
if done:
break
if len(agent.memory) > batch_size:
agent.replay(batch_size)
```
4. 运行CARLA仿真
```shell
./CarlaUE4.sh -windowed -carla-server -benchmark -fps=20
```
阅读全文
相关推荐
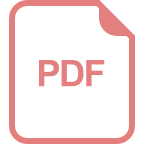
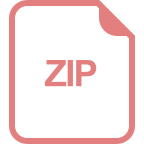
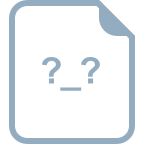
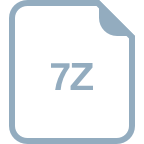
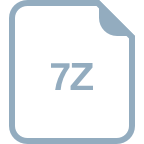
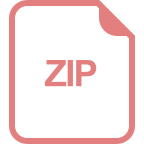
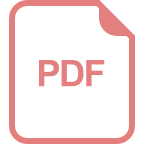
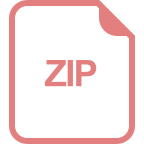
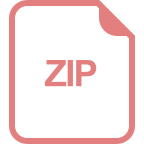
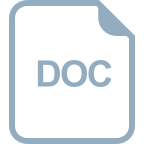
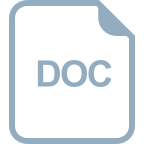
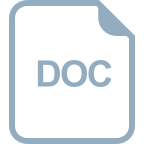
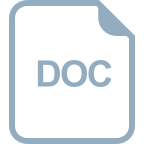
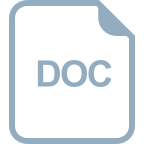
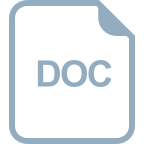