遗传算法多目标优化,请非常例子并生成代码
时间: 2024-09-10 20:19:01 浏览: 57
遗传算法是一种模拟自然选择和遗传学原理的搜索启发式算法,它被广泛用于求解优化问题。多目标优化指的是同时对多个目标进行优化的情况。在多目标优化问题中,通常不存在一个单一解可以同时优化所有目标,因此目标之间的权衡变得非常重要。遗传算法在多目标优化中的应用通常被称为多目标遗传算法(MOGA),它可以帮助找到一组解,这些解在多个目标之间提供了权衡,也就是所谓的Pareto最优解集。
下面我们以一个简单的双目标优化问题为例:假设我们有两个目标,分别是最小化两个变量x和y的平方和,即min f1(x, y) = x^2 + y^2 和 min f2(x, y) = (x - 2)^2 + (y - 2)^2。我们希望通过遗传算法找到一组解,这组解中的每个解都在两个目标之间提供了权衡。
下面是一个使用Python编写的简化版多目标遗传算法的代码示例:
```python
import numpy as np
# 定义目标函数
def f1(x, y):
return x**2 + y**2
def f2(x, y):
return (x - 2)**2 + (y - 2)**2
# 定义个体类
class Individual:
def __init__(self, x, y):
self.x = x
self.y = y
self.fitness = np.array([f1(x, y), f2(x, y)])
# 初始化种群
def init_population(pop_size, x_range, y_range):
return [Individual(np.random.uniform(*x_range), np.random.uniform(*y_range)) for _ in range(pop_size)]
# 选择函数
def select(population, k):
# 使用Pareto排序选择
pareto_front = non_dominated_sorting(population)
selected = []
for front in pareto_front:
if len(selected) + len(front) <= k:
selected.extend(front)
else:
# 选择非支配层的个体,如果个体数超过所需数量,则按拥挤距离选择
crowding_distance = np.array([np.sum(np.abs(ind.fitness - ind2.fitness)) for ind in front])
sorted_front = np.argsort(crowding_distance)[::-1]
selected.extend([front[i] for i in sorted_front[:k - len(selected)]])
break
return selected
# 非支配排序函数
def non_dominated_sorting(population):
# 这里为了简化,我们不展示完整的非支配排序实现
pass
# 交叉和变异函数
def crossover(parent1, parent2):
# 这里为了简化,我们不展示具体的交叉实现
pass
def mutate(individual):
# 这里为了简化,我们不展示具体的变异实现
pass
# 遗传算法主函数
def genetic_algorithm(pop_size, x_range, y_range, generations):
population = init_population(pop_size, x_range, y_range)
for generation in range(generations):
selected = select(population, pop_size)
children = []
for i in range(0, pop_size, 2):
parent1, parent2 = selected[i], selected[i+1]
child1, child2 = crossover(parent1, parent2)
mutate(child1)
mutate(child2)
children.append(child1)
children.append(child2)
population = children
return population
# 运行遗传算法
pop_size = 100
x_range = (-10, 10)
y_range = (-10, 10)
generations = 50
best_solutions = genetic_algorithm(pop_size, x_range, y_range, generations)
```
请注意,上述代码中的非支配排序、交叉和变异函数仅提供了框架和注释,具体实现没有给出。在实际应用中,需要根据具体问题来详细实现这些函数。
阅读全文
相关推荐










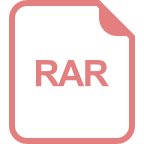






