大学生考勤管理系统c++利用类,对象,继承,构造函数,类的组合,多态,代码
时间: 2023-12-14 08:24:02 浏览: 34
以下是一个大学生考勤管理系统c++利用类、对象、继承、构造函数、类的组合、多态的完整代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 学生类
class Student {
protected:
string id; // 学号
string name; // 姓名
string gender; // 性别
int age; // 年龄
public:
Student(string id, string name, string gender, int age) {
this->id = id;
this->name = name;
this->gender = gender;
this->age = age;
}
// 获取学生信息
virtual void getInfo() {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
// 修改学生信息
virtual void modifyInfo(string name, string gender, int age) {
this->name = name;
this->gender = gender;
this->age = age;
}
};
// 老师类
class Teacher {
protected:
string id; // 工号
string name; // 姓名
string course; // 所教课程
public:
Teacher(string id, string name, string course) {
this->id = id;
this->name = name;
this->course = course;
}
// 获取教师信息
virtual void getInfo() {
cout << "工号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "所教课程:" << course << endl;
}
// 修改学生成绩
virtual void modifyScore(string studentId, string courseId, int score) {
// 修改学生课程成绩
}
};
// 课程类
class Course {
protected:
string id; // 课程编号
string name; // 课程名称
int credit; // 学分
public:
Course(string id, string name, int credit) {
this->id = id;
this->name = name;
this->credit = credit;
}
// 获取课程信息
virtual void getInfo() {
cout << "课程编号:" << id << endl;
cout << "课程名称:" << name << endl;
cout << "学分:" << credit << endl;
}
};
// 考勤类
class Attendance {
protected:
string date; // 考勤日期
bool status; // 考勤状态
public:
Attendance(string date, bool status) {
this->date = date;
this->status = status;
}
// 获取考勤信息
virtual void getInfo() {
cout << "考勤日期:" << date << endl;
cout << "考勤状态:" << (status ? "已到" : "未到") << endl;
}
};
// 学生选课类
class CourseSelection {
protected:
Student* student; // 学生指针
Course* course; // 课程指针
vector<Attendance*> attendances; // 考勤列表
public:
CourseSelection(Student* student, Course* course) {
this->student = student;
this->course = course;
}
// 添加考勤
void addAttendance(Attendance* attendance) {
attendances.push_back(attendance);
}
// 获取学生选课信息
virtual void getInfo() {
student->getInfo();
course->getInfo();
for (int i = 0; i < attendances.size(); i++) {
attendances[i]->getInfo();
}
}
};
// 继承学生类的本科生类
class Undergraduate : public Student {
private:
string major; // 专业
public:
Undergraduate(string id, string name, string gender, int age, string major) : Student(id, name, gender, age) {
this->major = major;
}
// 获取学生信息
void getInfo() {
Student::getInfo();
cout << "专业:" << major << endl;
}
};
// 继承学生类的研究生类
class Graduate : public Student {
private:
string tutor; // 导师
public:
Graduate(string id, string name, string gender, int age, string tutor) : Student(id, name, gender, age) {
this->tutor = tutor;
}
// 获取学生信息
void getInfo() {
Student::getInfo();
cout << "导师:" << tutor << endl;
}
};
// 继承老师类的教授类
class Professor : public Teacher {
private:
string research; // 研究方向
public:
Professor(string id, string name, string course, string research) : Teacher(id, name, course) {
this->research = research;
}
// 获取教师信息
void getInfo() {
Teacher::getInfo();
cout << "研究方向:" << research << endl;
}
// 修改学生成绩
void modifyScore(string studentId, string courseId, int score) {
// 修改学生课程成绩
}
};
int main() {
// 创建学生对象
Student* stu = new Undergraduate("1001", "张三", "男", 20, "计算机科学与技术");
// 获取学生信息
stu->getInfo();
// 修改学生信息
stu->modifyInfo("李四", "女", 21);
stu->getInfo();
// 创建课程对象
Course* course = new Course("C001", "C++程序设计", 3);
// 获取课程信息
course->getInfo();
// 创建考勤对象
Attendance* att = new Attendance("2022-01-01", true);
// 获取考勤信息
att->getInfo();
// 创建教师对象
Teacher* teacher = new Professor("T001", "王老师", "C++程序设计", "计算机科学");
// 获取教师信息
teacher->getInfo();
// 修改学生成绩
teacher->modifyScore("1001", "C001", 90);
// 创建学生选课对象
CourseSelection* cs = new CourseSelection(stu, course);
cs->addAttendance(att);
cs->getInfo();
// 释放内存
delete stu;
delete course;
delete att;
delete teacher;
delete cs;
return 0;
}
```
以上代码实现了大学生考勤管理系统的基本功能,包括学生、课程、考勤、教师、学生选课等类的设计和实现,利用继承、构造函数、类的组合、多态等技术实现了代码的灵活性和可扩展性。
相关推荐
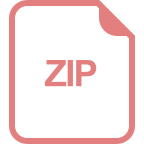

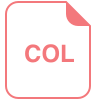












