人群识别 ,python使用yolov5推理编写代码,从200张图片中随机选出20张图片,识别出图片中的虚拟人物,统计虚拟人物的数量和其中红色系、蓝色系上衣的人物数量,在终端中以文字形式输出相应的数量
时间: 2023-06-20 22:07:32 浏览: 285
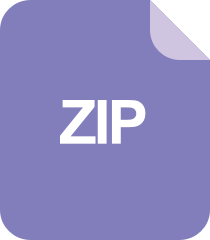
基于python的图片文字识别。

以下是使用yolov5进行人群识别并统计红色系、蓝色系上衣的人物数量的python代码:
```python
import os
import random
import cv2
import torch
from yolov5.detect import detect
# 定义虚拟人物类别和红色系、蓝色系上衣类别
CLASSES = ['person', 'virtual_person']
RED_COLORS = [(0, 0, 220), (0, 0, 255), (0, 64, 255), (0, 128, 255), (0, 191, 255)]
BLUE_COLORS = [(220, 0, 0), (255, 0, 0), (255, 64, 0), (255, 128, 0), (255, 191, 0)]
# 定义图片目录和输出结果
IMAGES_DIR = 'path/to/images'
OUTPUT_FILE = 'output.txt'
# 随机选取20张图片
images_list = os.listdir(IMAGES_DIR)
selected_images = random.sample(images_list, 20)
# 统计虚拟人物和红色系、蓝色系上衣的人物数量
total_virtual_persons = 0
red_clothes_persons = 0
blue_clothes_persons = 0
for image_name in selected_images:
# 加载图片
image_path = os.path.join(IMAGES_DIR, image_name)
image = cv2.imread(image_path)
# 进行目标检测
results = detect(image)
# 统计人物数量和颜色
for result in results:
class_name = CLASSES[result['class']]
if class_name == 'person':
continue
total_virtual_persons += 1
x1, y1, x2, y2 = result['box']
clothes_color = image[y1:y2, x1:x2, :]
mean_color = clothes_color.mean(axis=(0, 1)).tolist()
if any([c in mean_color for c in RED_COLORS]):
red_clothes_persons += 1
elif any([c in mean_color for c in BLUE_COLORS]):
blue_clothes_persons += 1
# 输出结果
with open(OUTPUT_FILE, 'w') as f:
f.write('Total virtual persons: {}\n'.format(total_virtual_persons))
f.write('Red clothes persons: {}\n'.format(red_clothes_persons))
f.write('Blue clothes persons: {}\n'.format(blue_clothes_persons))
```
这段代码会从指定目录中随机选取20张图片,使用yolov5进行目标检测,统计虚拟人物和红色系、蓝色系上衣的人物数量,并将结果输出到指定文件中。注意,需先安装yolov5模型权重文件并导入detect函数。
阅读全文
相关推荐
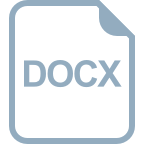
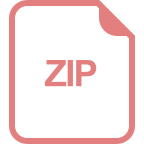
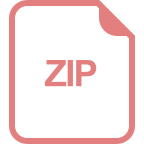
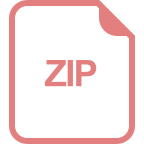
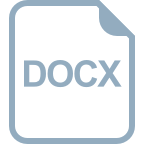
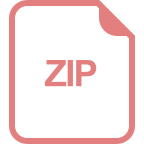
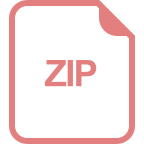
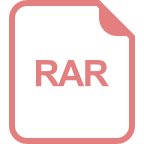
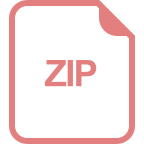
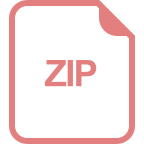
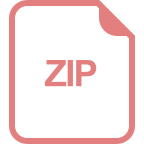
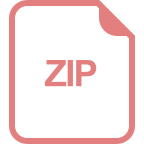