python线性拟合多条直线
时间: 2023-10-02 12:13:04 浏览: 242
要进行python线性拟合多条直线,可以使用最小二乘法来实现。首先,需要定义一个线性回归函数,用于计算每条直线的斜率和截距。然后,根据给定的数据点集,调用线性回归函数来计算每条直线的斜率和截距。最后,根据得到的斜率和截距,绘制出相应的直线。
下面是实现的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义线性回归函数
def linear_regression(x, y):
N = len(x)
sumx = sum(x)
sumy = sum(y)
sumx2 = sum(x**2)
sumxy = sum(x*y)
A = np.mat([[N, sumx], [sumx, sumx2]])
b = np.array([sumy, sumxy])
return np.linalg.solve(A, b)
# 定义数据点集
X = np.array([0, 1.34, 2.25, 4.67, 7.2, 9.6, 12.79, 15.61])
Y = np.array([0, 12.5, 25, 50, 100, 200, 400, 800])
# 定义要拟合的直线数量
num_lines = 3
# 进行线性拟合
lines = []
for i in range(num_lines):
start_index = i * len(X) // num_lines
end_index = (i + 1) * len(X) // num_lines
x_subset = X[start_index:end_index]
y_subset = Y[start_index:end_index]
a, b = linear_regression(x_subset, y_subset)
lines.append((a, b))
# 绘制拟合直线
_X = np.arange(0, 20, 0.01)
plt.figure(figsize=(10, 6))
plt.plot(X, Y, 'o', markersize=10, label='数据点')
for line in lines:
_Y = np.array([line[0] + line[1] * x for x in _X])
plt.plot(_X, _Y, linewidth=2, label='拟合直线')
plt.legend(fontsize=16, frameon=False)
plt.show()
```
阅读全文
相关推荐
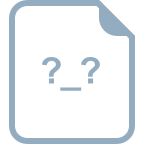
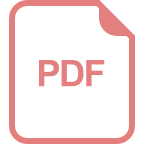

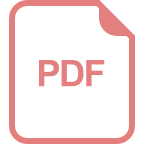
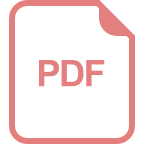
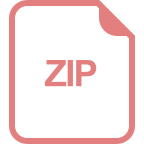


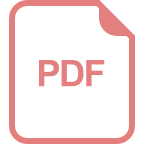
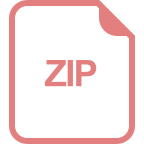
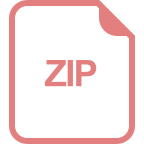
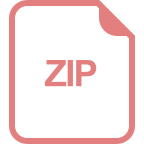
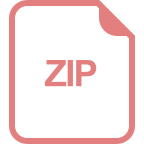
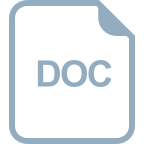
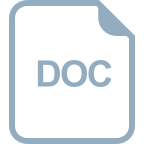
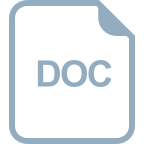
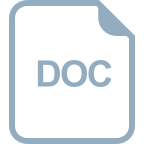
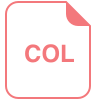