由n个点拟合多条直线python
时间: 2024-09-30 12:10:22 浏览: 39
在Python中,如果你想要通过n个点拟合多条直线,通常可以使用线性回归(Linear Regression)作为基础方法,特别是当这些点分布在多维空间并希望找到每个维度上独立的直线关系时。例如,你可以使用`numpy`库结合`scikit-learn`库中的`LinearRegression`模型。下面是一个简单的示例:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
# 假设你有n个二维数据点,每行代表一个点,最后一列是目标值
data = np.array([[x1, y1, target1], [x2, y2, target2], ..., [xn, yn, targetn]])
points = data[:, :-1]
targets = data[:, -1]
# 创建并训练线性回归模型
model = LinearRegression()
model.fit(points, targets)
# 对新的点预测
new_points = np.array([[new_x1, new_y1], [new_x2, new_y2], ...])
predicted_targets = model.predict(new_points)
```
在这个例子中,`model.coef_`将给出每一条直线上对应坐标轴的斜率,而`model.intercept_`则给出了y轴截距。
相关问题
python线性拟合多条直线
要进行python线性拟合多条直线,可以使用最小二乘法来实现。首先,需要定义一个线性回归函数,用于计算每条直线的斜率和截距。然后,根据给定的数据点集,调用线性回归函数来计算每条直线的斜率和截距。最后,根据得到的斜率和截距,绘制出相应的直线。
下面是实现的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义线性回归函数
def linear_regression(x, y):
N = len(x)
sumx = sum(x)
sumy = sum(y)
sumx2 = sum(x**2)
sumxy = sum(x*y)
A = np.mat([[N, sumx], [sumx, sumx2]])
b = np.array([sumy, sumxy])
return np.linalg.solve(A, b)
# 定义数据点集
X = np.array([0, 1.34, 2.25, 4.67, 7.2, 9.6, 12.79, 15.61])
Y = np.array([0, 12.5, 25, 50, 100, 200, 400, 800])
# 定义要拟合的直线数量
num_lines = 3
# 进行线性拟合
lines = []
for i in range(num_lines):
start_index = i * len(X) // num_lines
end_index = (i + 1) * len(X) // num_lines
x_subset = X[start_index:end_index]
y_subset = Y[start_index:end_index]
a, b = linear_regression(x_subset, y_subset)
lines.append((a, b))
# 绘制拟合直线
_X = np.arange(0, 20, 0.01)
plt.figure(figsize=(10, 6))
plt.plot(X, Y, 'o', markersize=10, label='数据点')
for line in lines:
_Y = np.array([line[0] + line[1] * x for x in _X])
plt.plot(_X, _Y, linewidth=2, label='拟合直线')
plt.legend(fontsize=16, frameon=False)
plt.show()
```
如何实现多条直线拟合python
实现多条直线拟合可以使用Python中的Scikit-learn库。具体步骤如下:
1. 导入所需库和数据
```python
from sklearn.linear_model import LinearRegression
import numpy as np
# 定义数据
X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]])
y = np.dot(X, np.array([1, 2])) + 3
```
2. 定义模型
```python
# 定义模型
model = LinearRegression()
```
3. 拟合数据
```python
# 拟合数据
model.fit(X, y)
```
4. 预测结果
```python
# 预测结果
model.predict(np.array([[3, 5]]))
```
对于多条直线的拟合,可以将数据集分成多个子集,每个子集拟合一条直线,然后将这些直线拼接起来形成一条完整的直线。具体实现可以按照以下步骤:
1. 将数据按照x轴坐标进行排序
```python
data = sorted(data, key=lambda x:x[0])
```
2. 将数据分成多个子集
```python
subsets = []
subset = []
for i in range(len(data)-1):
subset.append(data[i])
if data[i+1][0] > data[i][0] + threshold:
subsets.append(subset)
subset = []
subsets.append(subset)
```
其中,threshold是一个阈值,用于判断两个点是否属于同一子集。
3. 对每个子集进行拟合
```python
models = []
for subset in subsets:
X = np.array([x[0] for x in subset]).reshape(-1, 1)
y = np.array([x[1] for x in subset]).reshape(-1, 1)
model = LinearRegression()
model.fit(X, y)
models.append(model)
```
4. 将所有直线拼接起来形成一条完整的直线
```python
def predict(x):
for model in models:
if x < model.intercept_:
return model.predict([[x]])[0][0]
return models[-1].predict([[x]])[0][0]
```
阅读全文
相关推荐
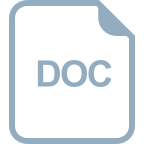
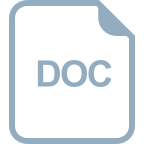
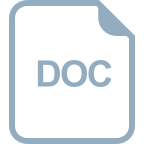

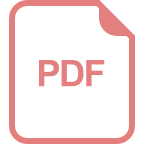
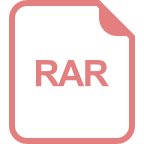










