ava jgit实现pull代码到本地
时间: 2024-05-07 11:21:32 浏览: 12
使用 Java JGit 可以很方便地实现代码的 pull 操作,具体步骤如下:
1. 引入 JGit 依赖
在 Maven 项目中,可以在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.eclipse.jgit</groupId>
<artifactId>org.eclipse.jgit</artifactId>
<version>5.12.0.201906051850-r</version>
</dependency>
```
2. 创建 Git 对象
```java
Git git = Git.open(new File("/path/to/your/local/repo"));
```
其中,`/path/to/your/local/repo` 是你本地代码仓库的路径。
3. 设置 PullCommand
```java
PullCommand pullCmd = git.pull();
```
4. 设置 PullCommand 的参数
```java
pullCmd.setRemote("origin"); // 远程仓库名称
pullCmd.setRemoteBranchName("master"); // 远程分支名称
pullCmd.setCredentialsProvider(new UsernamePasswordCredentialsProvider("username", "password")); // 认证信息,可选
```
其中,`username` 和 `password` 分别是你的 Git 账号和密码。
5. 执行 PullCommand
```java
pullCmd.call();
```
执行完毕后,代码就会被拉取到本地仓库。完整代码示例:
```java
import java.io.File;
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.PullCommand;
import org.eclipse.jgit.transport.UsernamePasswordCredentialsProvider;
public class GitPullDemo {
public static void main(String[] args) throws Exception {
Git git = Git.open(new File("/path/to/your/local/repo"));
PullCommand pullCmd = git.pull();
pullCmd.setRemote("origin");
pullCmd.setRemoteBranchName("master");
pullCmd.setCredentialsProvider(new UsernamePasswordCredentialsProvider("username", "password"));
pullCmd.call();
}
}
```
注意:在执行 PullCommand 之前,应该先确保本地仓库是干净的(即没有未提交的修改)。如果有未提交的修改,可以使用以下代码进行提交:
```java
git.add().addFilepattern(".").call();
git.commit().setMessage("commit message").call();
```
其中,`commit message` 是提交信息。
相关推荐
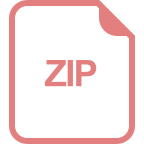














