用jsp写一个员工设置时间打卡的代码
时间: 2024-03-07 14:48:05 浏览: 25
好的,下面是一个简单的员工设置时间打卡的 JSP 代码示例:
1. 创建一个名为 `login.jsp` 的登录页面,在其中添加一个表单,用于输入员工的用户名和密码:
```html
<form action="checkLogin.jsp" method="post">
<label for="username">用户名:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">密码:</label>
<input type="password" id="password" name="password">
<br>
<input type="submit" value="登录">
</form>
```
2. 创建一个名为 `checkLogin.jsp` 的 JSP 页面,用于验证员工的用户名和密码,如果验证通过,则跳转到打卡时间设置页面:
```jsp
<%
// 获取用户输入的用户名和密码
String username = request.getParameter("username");
String password = request.getParameter("password");
// 假设用户名和密码为 admin/admin
if ("admin".equals(username) && "admin".equals(password)) {
// 验证通过,跳转到打卡时间设置页面
response.sendRedirect("setTime.jsp");
} else {
// 验证失败,返回登录页面
response.sendRedirect("login.jsp");
}
%>
```
3. 创建一个名为 `setTime.jsp` 的 JSP 页面,用于设置员工的打卡时间。在该页面中,添加一个表单,用于输入员工的打卡时间:
```html
<form action="saveTime.jsp" method="post">
<label for="time">打卡时间:</label>
<input type="time" id="time" name="time">
<br>
<input type="submit" value="保存">
</form>
```
4. 创建一个名为 `saveTime.jsp` 的 JSP 页面,用于保存员工的打卡时间。在该页面中,获取用户输入的打卡时间,并将其保存到数据库中:
```jsp
<%@ page import="java.sql.*" %>
<%@ page import="java.time.LocalTime" %>
<%
// 获取用户输入的打卡时间
String timeStr = request.getParameter("time");
LocalTime time = LocalTime.parse(timeStr);
// 假设数据库中已经创建了一张名为 "employee" 的表,其中包含 "id" 和 "time" 两个字段
// 创建与数据库的连接
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, username, password);
// 执行 SQL 语句,将打卡时间保存到数据库中
String sql = "UPDATE employee SET time=? WHERE id=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setTime(1, Time.valueOf(time));
pstmt.setInt(2, 1); // 假设员工的 id 为 1
pstmt.executeUpdate();
// 关闭数据库连接
pstmt.close();
conn.close();
%>
<p>打卡时间保存成功!</p>
```
5. 最后,创建一个名为 `checkTime.jsp` 的 JSP 页面,用于检查员工的打卡时间是否已经到达。在该页面中,从数据库中查询员工的打卡时间,并与当前时间进行比较:
```jsp
<%@ page import="java.sql.*" %>
<%@ page import="java.time.LocalTime" %>
<%
// 从数据库中查询员工的打卡时间
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, username, password);
String sql = "SELECT time FROM employee WHERE id=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, 1); // 假设员工的 id 为 1
ResultSet rs = pstmt.executeQuery();
LocalTime time = null;
if (rs.next()) {
time = rs.getTime("time").toLocalTime();
}
// 关闭数据库连接
rs.close();
pstmt.close();
conn.close();
// 比较时间
if (time != null) {
LocalTime now = LocalTime.now();
if (now.isAfter(time)) {
// 已经到了打卡时间
out.println("已经到了打卡时间!");
} else {
// 还未到打卡时间
out.println("还未到打卡时间!");
}
} else {
out.println("无法获取打卡时间!");
}
%>
```
以上就是一个简单的员工设置时间打卡的 JSP 代码示例,具体实现需要根据实际情况进行调整。另外,为了安全起见,建议在生产环境中使用加密算法对密码进行加密。
相关推荐
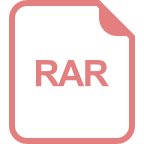
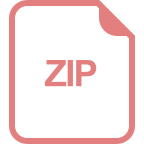
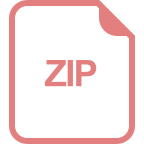














