用jsp代码写一个数据库验证登陆系统
时间: 2023-12-31 19:06:45 浏览: 29
以下是一个简单的用JSP代码实现的数据库验证登录系统的例子:
login.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<% String message = (String)request.getAttribute("message"); %>
<% if (message != null) { %>
<p style="color: red;"><%= message %></p>
<% } %>
<form action="login.do" method="post">
Username: <input type="text" name="username"><br>
Password: <input type="password" name="password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
login.do:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%@ page import="javax.sql.*" %>
<%@ page import="javax.naming.*" %>
<%
String username = request.getParameter("username");
String password = request.getParameter("password");
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
Context initContext = new InitialContext();
Context envContext = (Context)initContext.lookup("java:/comp/env");
DataSource ds = (DataSource)envContext.lookup("jdbc/mydb");
conn = ds.getConnection();
stmt = conn.prepareStatement("SELECT * FROM users WHERE username = ? AND password = ?");
stmt.setString(1, username);
stmt.setString(2, password);
rs = stmt.executeQuery();
if (rs.next()) {
session.setAttribute("username", username);
response.sendRedirect("welcome.jsp");
} else {
request.setAttribute("message", "Invalid username or password");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
} catch (Exception e) {
e.printStackTrace();
request.setAttribute("message", "Database error");
request.getRequestDispatcher("login.jsp").forward(request, response);
} finally {
if (rs != null) {
try { rs.close(); } catch (SQLException e) { }
}
if (stmt != null) {
try { stmt.close(); } catch (SQLException e) { }
}
if (conn != null) {
try { conn.close(); } catch (SQLException e) { }
}
}
%>
```
welcome.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Welcome</title>
</head>
<body>
<h1>Welcome <%= session.getAttribute("username") %>!</h1>
<a href="logout.do">Logout</a>
</body>
</html>
```
logout.do:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
session.invalidate();
response.sendRedirect("login.jsp");
%>
```
以上代码使用了JNDI数据源连接数据库,并通过PreparedStatement和ResultSet验证用户名和密码。如果验证成功,将用户名保存到session中,并重定向到welcome.jsp页面。如果验证失败,则将错误消息保存到request中,并转发回login.jsp页面。登出时,通过session.invalidate()方法使session失效,并重定向回login.jsp页面。
相关推荐
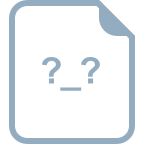














