在http://www.tianqihoubao.com网站上选择一个城市(石家庄除外),利用Python编写网络爬虫爬取该城市过去一个月的天气情况,并按照时间正序进行输出。
时间: 2024-06-08 22:07:37 浏览: 8
好的,我可以教你如何使用 Python 进行网络爬虫并抓取网页上的天气信息。首先,你需要安装 requests 和 BeautifulSoup 库,可以通过以下命令进行安装:
```python
pip install requests
pip install beautifulsoup4
```
接下来,你可以按照以下步骤编写代码:
1. 导入所需的库:
```python
import requests
from bs4 import BeautifulSoup
```
2. 定义要爬取的城市和时间范围:
```python
city = 'beijing'
start_date = '2022-01-01'
end_date = '2022-01-31'
```
3. 构造 URL 和请求头:
```python
url = f'http://www.tianqihoubao.com/lishi/{city}/month/{start_date}.html'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
```
4. 发送请求并解析网页:
```python
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
```
5. 定位天气信息所在的表格,并将其转化为二维数组:
```python
table = soup.find('table', class_='b')
rows = table.find_all('tr')[1:]
data = [[td.text.strip() for td in tr.find_all('td')] for tr in rows]
```
6. 过滤出指定日期范围内的天气信息:
```python
filtered_data = [row for row in data if start_date <= row[0] <= end_date]
```
7. 按照时间正序进行输出:
```python
for row in filtered_data:
print(row[0], row[1], row[2], row[3], row[4])
```
完整代码如下:
```python
import requests
from bs4 import BeautifulSoup
city = 'beijing'
start_date = '2022-01-01'
end_date = '2022-01-31'
url = f'http://www.tianqihoubao.com/lishi/{city}/month/{start_date}.html'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
table = soup.find('table', class_='b')
rows = table.find_all('tr')[1:]
data = [[td.text.strip() for td in tr.find_all('td')] for tr in rows]
filtered_data = [row for row in data if start_date <= row[0] <= end_date]
for row in filtered_data:
print(row[0], row[1], row[2], row[3], row[4])
```
你只需要将代码中的城市名和时间范围修改为你需要的就可以了。
相关推荐
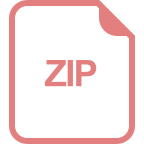
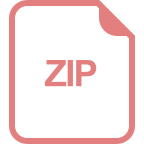
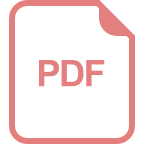














