import pandas as pd import matplotlib.pyplot as plt from sklearn.model_selection import train_test_split # 数据分析 df = pd.read_csv('iris.csv', skiprows=[0], names=['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'class']) print(df.info()) print(df.describe()) print(df.isnull().sum()) # 随机抽取数据 train_data, test_data = train_test_split(df, test_size=0.3) train_data.to_csv('train_data.csv', index=False) test_data.to_csv('test_data.csv', index=False) # 数据可视化 df.plot(x='class', y=['sepal_length', 'sepal_width', 'petal_length', 'petal_width'], kind='line', title='Line Plot', figsize=(10, 5)) df.hist(bins=10, figsize=(10, 5)) df.plot(x='petal_length', y='petal_width', kind='scatter', title='Scatter Plot', figsize=(10, 5)) plt.show() 对上面每一行代码注释
时间: 2023-09-23 09:04:00 浏览: 64
Sure, here are the explanations for each line of code:
```
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
```
这里是导入所需的库,分别是 pandas、matplotlib 和 sklearn 中的 train_test_split 函数。
```
df = pd.read_csv('iris.csv', skiprows=[0], names=['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'class'])
```
这里使用 pandas 库中的 read_csv() 方法读取名为 iris.csv 的文件,skiprows=[0] 表示跳过第一行,names 参数指定了每一列的名称。
```
print(df.info())
print(df.describe())
print(df.isnull().sum())
```
这里分别使用了 info()、describe() 和 isnull() 方法对数据进行统计分析,包括数据的维度、每个属性的均值、标准差、最小值、最大值、缺失值数量等等。
```
train_data, test_data = train_test_split(df, test_size=0.3)
train_data.to_csv('train_data.csv', index=False)
test_data.to_csv('test_data.csv', index=False)
```
这里使用了 sklearn 中的 train_test_split() 方法将数据集划分为训练集和测试集,并将它们保存到 train_data.csv 和 test_data.csv 文件中。
```
df.plot(x='class', y=['sepal_length', 'sepal_width', 'petal_length', 'petal_width'], kind='line', title='Line Plot', figsize=(10, 5))
df.hist(bins=10, figsize=(10, 5))
df.plot(x='petal_length', y='petal_width', kind='scatter', title='Scatter Plot', figsize=(10, 5))
plt.show()
```
这里使用了 matplotlib 库对数据进行可视化,包括了折线图、直方图和散点图。这些可视化展示了不同属性之间的关系,方便我们更好地理解和分析数据。最后使用 plt.show() 方法显示图形。
相关推荐
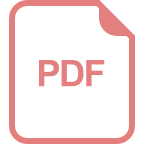














