帮我把3在重新详细写一下
时间: 2024-10-23 19:02:52 浏览: 6
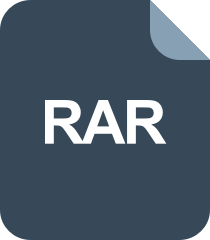
CC3D的两大飞行模式的原理详细说明
### 实验三 - 定义抽象类 `Animal` 和类 `Dog`
#### 类 `Animal` 的定义
1. **参数列表**:
- `name: String`: 动物的名字。
- `age: Int`: 动物的年龄。
- 这些参数使用 `val` 修饰符,表示它们是不可变的。
2. **主构造器**:
- 在主构造器中打印 `"This is the superclass constructor"`。
3. **静态工厂方法 `apply()`**:
- 定义一个静态工厂方法 `apply()`,打印 `"this is Animal."`。
4. **抽象方法 `Action()`**:
- 定义一个抽象方法 `Action()`,由子类实现。
5. **具体方法 `hair()`**:
- 定义一个具体方法 `hair()`,打印 `"has hair."`。
```scala
abstract class Animal(val name: String, val age: Int) {
println("This is the superclass constructor")
def Action(): Unit
def hair(): Unit = {
println(s"$name has hair.")
}
def apply(): Unit = {
println("this is Animal.")
}
}
```
#### 类 `Dog` 的定义
1. **继承 `Animal`**:
- 继承 `Animal` 类,并在其参数列表基础上增加一个 `sound: String` 参数,表示狗的叫声。
- 不实现 `sound` 的 setter 和 getter 方法。
2. **主构造器**:
- 在主构造器中打印 `"This is the subclass primary constructor"`。
3. **辅助构造器**:
- 添加一个辅助构造器,额外接受一个 `smell: String` 参数,表示狗的嗅觉能力。
- 在辅助构造器中打印 `"I have a dog named $name, who is $age years old and can $sound. His nose is very sensitive and can $smell."`。
4. **重写方法**:
- 重写 `hair()` 方法,打印 `"$name has hair."`。
- 重写 `Action()` 方法,打印 `"$name can jump."`。
5. **成员字段 `ID`**:
- 定义一个成员字段 `ID`,通过调用伴生对象中的 `CurrentID()` 方法实现赋值。
- 提供一个 `printID()` 方法,用于打印 `ID` 值。
6. **静态工厂方法 `apply(sound: String)`**:
- 定义一个静态工厂方法 `apply(sound: String)`,用于重新设置 `sound` 并打印 `"The $age-year-old $name's bark became $sound."`。
```scala
class Dog(name: String, age: Int, sound: String) extends Animal(name, age) {
println("This is the subclass primary constructor")
def this(name: String, age: Int, sound: String, smell: String) {
this(name, age, sound)
println(s"I have a dog named $name, who is $age years old and can $sound. His nose is very sensitive and can $smell.")
}
override def hair(): Unit = {
println(s"$name has hair.")
}
override def Action(): Unit = {
println(s"$name can jump.")
}
var ID: Int = Dog.CurrentID()
def printID(): Unit = {
println(s"The dog's ID is $ID")
}
def apply(newSound: String): Unit = {
this.sound = newSound
println(s"The $age-year-old $name's bark became $newSound.")
}
}
object Dog {
private var ID: Int = 0
def CurrentID(): Int = {
ID += 1
ID
}
def apply(): Unit = {
println("this is object Dog.")
}
def apply(name: String, age: Int, sound: String): Dog = {
new Dog(name, age, sound)
}
}
```
#### 样例代码
1. **实例化 `Dog` 类**:
```scala
val dog1 = new Dog("Bob", 5, "WangWang")
```
2. **访问 `hair()`、`Action()`、`printID()` 方法**:
```scala
dog1.hair()
dog1.Action()
dog1.printID()
```
3. **调用 `apply()` 方法**:
```scala
dog1.apply("MiaoMiao")
```
4. **实例化带有辅助构造器的 `Dog` 类**:
```scala
val dog2 = new Dog("Tommi", 3, "WangWang", "smell")
```
5. **访问 `printID()` 方法**:
```scala
dog2.printID()
```
6. **直接改变 `sound` 的值**:
```scala
dog2.sound = "MiaoMiao"
println(dog2.sound)
```
7. **实例化 `Dog` 类并通过伴生对象的 `apply()` 方法**:
```scala
val dog3 = Dog("Max", 4, "Woof")
Dog.apply()
```
以上代码展示了如何定义抽象类 `Animal` 和类 `Dog`,包括继承、构造器、辅助构造器、方法重写、静态工厂方法和伴生对象的使用。
阅读全文
相关推荐
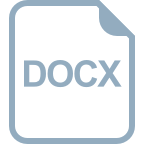
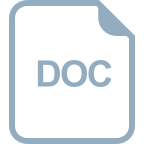















