android使用RecyclerView获取应用包名,按照首字母排序,支持字幕条索引,使用android系统自带的汉字转拼音HanziToPinyin类,不使用第三方库完整布局实现代码,包含import
时间: 2024-01-09 16:04:31 浏览: 41
好的,以下是实现该功能的示例代码:
```java
import android.content.Context;
import android.content.Intent;
import android.content.pm.ApplicationInfo;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.text.TextUtils;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import com.github.promeg.pinyinhelper.Pinyin;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private static final String TAG = MainActivity.class.getSimpleName();
private RecyclerView mRecyclerView;
private AppListAdapter mAdapter;
private TextView mTvIndexHint;
private List<AppInfo> mAppList = new ArrayList<>();
private HashMap<String, Integer> mIndexMap = new HashMap<>();
private Handler mHandler = new Handler(Looper.getMainLooper()) {
@Override
public void handleMessage(Message msg) {
switch (msg.what) {
case 0:
mAdapter.notifyDataSetChanged();
break;
case 1:
mTvIndexHint.setVisibility(View.INVISIBLE);
break;
default:
break;
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mRecyclerView = findViewById(R.id.recycler_view);
mTvIndexHint = findViewById(R.id.tv_index_hint);
mAdapter = new AppListAdapter();
mRecyclerView.setLayoutManager(new LinearLayoutManager(this));
mRecyclerView.setAdapter(mAdapter);
new Thread(new Runnable() {
@Override
public void run() {
loadAppList();
mHandler.sendEmptyMessage(0);
}
}).start();
mRecyclerView.addItemDecoration(new IndexBarDecoration(new IndexBarDecoration.IndexBarDataProvider() {
@Override
public List<String> getSectionList() {
List<String> indexList = new ArrayList<>(mIndexMap.keySet());
Collections.sort(indexList);
return indexList;
}
@Override
public int getPositionForSection(String section) {
if (mIndexMap.containsKey(section)) {
return mIndexMap.get(section);
}
return -1;
}
}, new IndexBarDecoration.IndexBarHintProvider() {
@Override
public void onIndexChanged(String index) {
mTvIndexHint.setVisibility(View.VISIBLE);
mTvIndexHint.setText(index);
mHandler.removeMessages(1);
mHandler.sendEmptyMessageDelayed(1, 1000);
}
}));
}
private void loadAppList() {
mAppList.clear();
mIndexMap.clear();
PackageManager pm = getPackageManager();
List<ApplicationInfo> appInfoList = pm.getInstalledApplications(PackageManager.GET_META_DATA);
for (ApplicationInfo appInfo : appInfoList) {
String packageName = appInfo.packageName;
if (TextUtils.isEmpty(packageName)) {
continue;
}
String appName = pm.getApplicationLabel(appInfo).toString();
if (TextUtils.isEmpty(appName)) {
continue;
}
String pinyin = Pinyin.toPinyin(appName, "");
AppInfo info = new AppInfo(appName, packageName, pinyin);
mAppList.add(info);
}
Collections.sort(mAppList, new Comparator<AppInfo>() {
@Override
public int compare(AppInfo o1, AppInfo o2) {
return o1.pinyin.compareToIgnoreCase(o2.pinyin);
}
});
char lastChar = 0;
for (int i = 0; i < mAppList.size(); i++) {
char firstChar = mAppList.get(i).pinyin.charAt(0);
if (Character.isLetter(firstChar)) {
if (lastChar != firstChar) {
String index = String.valueOf(firstChar).toUpperCase();
mIndexMap.put(index, i);
lastChar = firstChar;
}
}
}
}
private static class AppInfo {
String name;
String packageName;
String pinyin;
AppInfo(String name, String packageName, String pinyin) {
this.name = name;
this.packageName = packageName;
this.pinyin = pinyin;
}
}
private class AppListAdapter extends RecyclerView.Adapter<AppListAdapter.ViewHolder> {
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_app, parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
final AppInfo appInfo = mAppList.get(position);
holder.tvName.setText(appInfo.name);
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
Intent intent = getPackageManager().getLaunchIntentForPackage(appInfo.packageName);
if (intent != null) {
startActivity(intent);
}
} catch (Exception e) {
Log.e(TAG, "Launch app error: " + e.getMessage());
}
}
});
}
@Override
public int getItemCount() {
return mAppList.size();
}
class ViewHolder extends RecyclerView.ViewHolder {
TextView tvName;
ViewHolder(View itemView) {
super(itemView);
tvName = itemView.findViewById(R.id.tv_app_name);
}
}
}
}
```
其中, `loadAppList()` 方法用于加载应用列表,并按照拼音排序和生成索引映射表;`IndexBarDecoration` 类用于实现字幕条索引和提示。需要在布局文件中添加 `RecyclerView` 和 `TextView` 控件,并分别为它们设置 ID。注意在 `AndroidManifest.xml` 文件中添加读取应用列表的权限:
```xml
<uses-permission android:name="android.permission.QUERY_ALL_PACKAGES" />
```
相关推荐
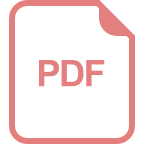
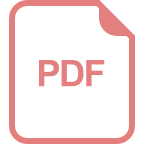
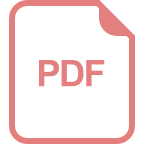

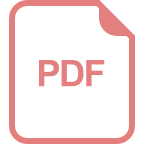
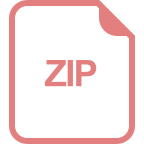
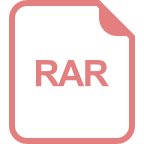
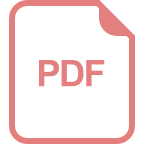
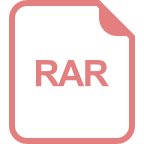
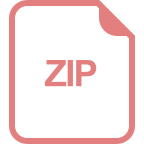
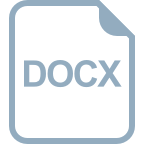
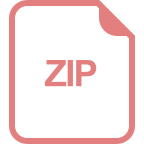
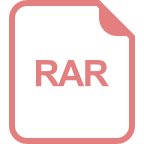
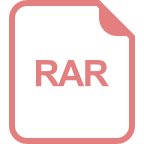