python爬取京东手机多页网页并连接数据库,将数据放进数据库,用pyecharts做数据可视化处理
时间: 2024-03-21 19:37:50 浏览: 16
好的,这是一个比较复杂的问题,我会尽力为您解答。首先,爬取京东手机多页网页需要使用Python的requests库和BeautifulSoup库。以下是示例代码:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='testdb', charset='utf8mb4')
cursor = conn.cursor()
# 爬取数据
for i in range(1, 11):
url = 'https://list.jd.com/list.html?cat=9987,653,655&page={}'.format(i)
r = requests.get(url)
soup = BeautifulSoup(r.text, 'html.parser')
# 解析数据
items = soup.find_all('li', class_='gl-item')
for item in items:
sku = item['data-sku']
name = item.select('.p-name em')[0].text.strip()
price = item.select('.p-price i')[0].text.strip()
shop = item.select('.p-shop a')[0].text.strip()
# 插入数据到数据库
sql = "INSERT INTO jd_phone (sku, name, price, shop) VALUES ('{}', '{}', '{}', '{}')".format(sku, name, price, shop)
cursor.execute(sql)
conn.commit()
# 关闭数据库连接
cursor.close()
conn.close()
```
上述代码会爬取京东手机分类的前10页数据,并将数据插入到名为`jd_phone`的MySQL数据库中。
接下来,使用pyecharts库进行数据可视化处理。以下是示例代码:
```python
from pyecharts import options as opts
from pyecharts.charts import Bar
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='testdb', charset='utf8mb4')
cursor = conn.cursor()
# 查询数据
cursor.execute("SELECT shop, COUNT(*) FROM jd_phone GROUP BY shop ORDER BY COUNT(*) DESC")
data = cursor.fetchall()
# 绘制柱状图
x_data = [d[0] for d in data]
y_data = [d[1] for d in data]
bar = (
Bar()
.add_xaxis(x_data)
.add_yaxis("手机数量", y_data)
.set_global_opts(title_opts=opts.TitleOpts(title="京东手机店铺销量排名"))
)
bar.render("jd_phone.html")
# 关闭数据库连接
cursor.close()
conn.close()
```
上述代码会从名为`jd_phone`的MySQL数据库中查询每个店铺的销售数量,并绘制出柱状图。最后,将图表保存为`jd_phone.html`文件。
希望上述代码对您有所帮助!
相关推荐
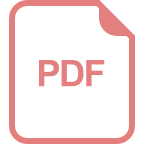
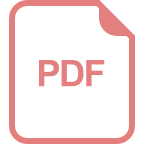














