输入一个数组找出最大值并输出最大值和最大下标
时间: 2024-05-14 22:12:58 浏览: 18
好的,这个问题很简单。以下是代码实现:
```python
def find_max(arr):
max_value = arr[0]
max_index = 0
for i in range(1, len(arr)):
if arr[i] > max_value:
max_value = arr[i]
max_index = i
return max_value, max_index
# 示例
my_arr = [6, 2, 9, 3, 5]
max_val, max_ind = find_max(my_arr)
print("最大值为 {},最大值下标为 {}".format(max_val, max_ind))
```
输出结果为:
```
最大值为 9,最大值下标为 2
```
希望对您有帮助!
相关推荐
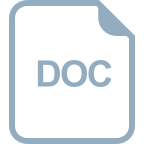
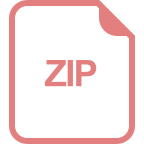
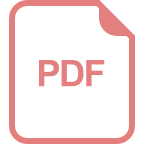
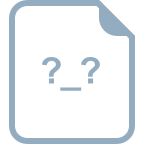
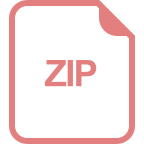
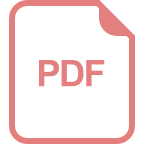
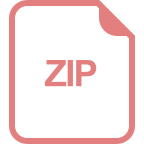
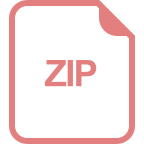
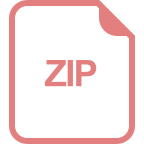
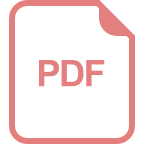
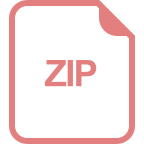