import numpy as np import pandas as pd import matplotlib.pyplot as plt import pyhht # 读取csv文件数据 data = pd.read_csv('77.csv', header=None) time = data.iloc[:, 0].values charge = data.iloc[:, 1].values # EMD分解 decomposer = pyhht.EMD(charge) imfs = decomposer.decompose() # 绘制原始序列和分解后的IMF分量 plt.figure(figsize=(10, 6)) plt.plot(time, charge, label='Original') plt.xlabel('Time') plt.ylabel('Charge') plt.legend() plt.show() for i in range(imfs.shape[0]): plt.plot(time, imfs[i], label=f'IMF{i+1}') plt.xlabel('Time') plt.ylabel('Charge') plt.legend() plt.show() residuals = charge - np.sum(imfs, axis=0) plt.plot(time, residuals, label='Residuals') plt.xlabel('Time') plt.ylabel('Charge') plt.legend() plt.show()在上述代码后增写一段代码,使得上述代码中绘制的图片可以显示在一张图中
时间: 2023-11-28 09:51:25 浏览: 39
可以使用 subplots() 方法将多个图像显示在同一个图中。以下是修改后的代码:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import pyhht
# 读取csv文件数据
data = pd.read_csv('77.csv', header=None)
time = data.iloc[:, 0].values
charge = data.iloc[:, 1].values
# EMD分解
decomposer = pyhht.EMD(charge)
imfs = decomposer.decompose()
# 绘制原始序列和分解后的IMF分量
fig, axs = plt.subplots(nrows=imfs.shape[0]+1, ncols=1, figsize=(10, 10))
axs[0].plot(time, charge, label='Original')
axs[0].set_xlabel('Time')
axs[0].set_ylabel('Charge')
axs[0].legend()
for i in range(imfs.shape[0]):
axs[i+1].plot(time, imfs[i], label=f'IMF{i+1}')
axs[i+1].set_xlabel('Time')
axs[i+1].set_ylabel('Charge')
axs[i+1].legend()
plt.tight_layout()
plt.show()
residuals = charge - np.sum(imfs, axis=0)
plt.plot(time, residuals, label='Residuals')
plt.xlabel('Time')
plt.ylabel('Charge')
plt.legend()
plt.show()
```
这样就可以将每个 IMF 和原始信号一起显示在同一个图中。`tight_layout()` 方法可以自动调整子图之间的间距,使它们更紧凑。
相关推荐
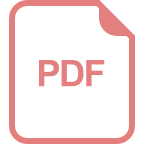
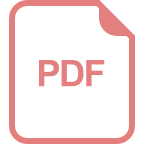
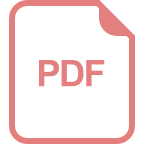















