用c++代码写一个滑雪游戏
时间: 2023-12-20 20:04:59 浏览: 156
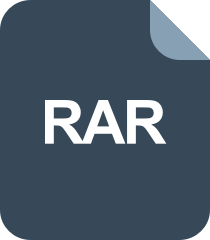
c++贪吃蛇游戏源代码.rar
以下是一个简单的滑雪游戏的C++代码示例:
```c++
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
// 游戏区域大小
const int WIDTH = 20;
const int HEIGHT = 10;
// 游戏角色类型
const char PLAYER_CHAR = 'P';
const char TREE_CHAR = 'T';
const char ROCK_CHAR = 'R';
// 游戏角色位置
int playerX = WIDTH / 2;
int playerY = HEIGHT - 1;
// 游戏角色状态
bool isAlive = true;
int score = 0;
// 随机生成游戏角色
char generateCharacter() {
int r = rand() % 10;
if (r < 3) {
return TREE_CHAR;
} else if (r < 6) {
return ROCK_CHAR;
} else {
return ' ';
}
}
// 打印游戏区域
void printGameArea() {
// 清空屏幕
system("cls");
// 打印游戏区域
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
if (x == playerX && y == playerY) {
cout << PLAYER_CHAR;
} else {
cout << generateCharacter();
}
}
cout << endl;
}
// 打印得分
cout << "Score: " << score << endl;
}
// 处理用户输入
void handleInput() {
if (GetAsyncKeyState(VK_LEFT) && playerX > 0) {
playerX--;
}
if (GetAsyncKeyState(VK_RIGHT) && playerX < WIDTH - 1) {
playerX++;
}
}
// 更新游戏状态
void updateGame() {
// 检测是否撞到障碍物
char c = generateCharacter();
if (c == TREE_CHAR || c == ROCK_CHAR) {
if (playerX == WIDTH / 2 && playerY == HEIGHT - 1) {
isAlive = false;
}
}
// 更新得分
score++;
}
int main() {
// 设置随机数种子
srand(time(NULL));
// 游戏主循环
while (isAlive) {
handleInput();
updateGame();
printGameArea();
// 暂停一段时间
Sleep(50);
}
// 打印游戏结束信息
cout << "Game Over! Your score is " << score << endl;
return 0;
}
```
上述代码使用了Windows API中的GetAsyncKeyState函数来检测用户输入,需要在Windows环境下编译运行。游戏区域大小、角色类型和位置等信息可以根据需要进行调整。
阅读全文
相关推荐
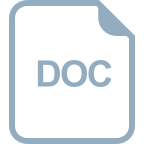
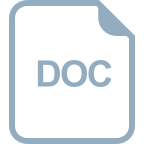
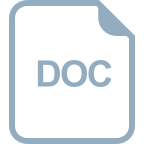
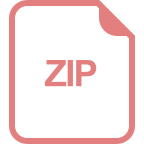
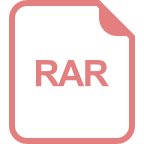
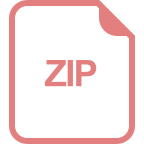
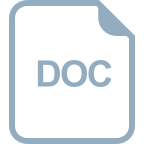
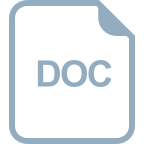
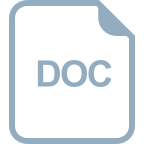
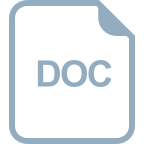
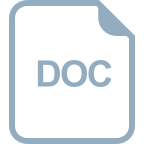
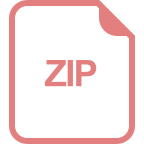
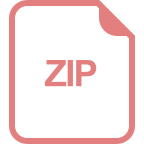
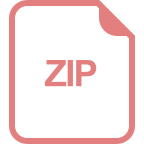
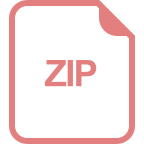