写一个python读取和写入数据例子
时间: 2023-03-14 07:03:53 浏览: 66
示例代码:# 导入 CSV 模块
import csv
# 将数据写入 CSV 文件
with open('data.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['name', 'age'])
writer.writerow(['Bob', '19'])
writer.writerow(['Tom', '20'])
# 从 CSV 文件读取数据
with open('data.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
相关问题
python读取和写入txt文件
Python提供了许多方法来读写文本文件。其中最常用的方法是使用open()函数打开文件,然后使用read()或write()方法读取或写入文件内容。
以下是一个简单的例子,演示了如何读取和写入文本文件:
读取文件:
```python
with open('filename.txt', 'r') as file:
data = file.read()
print(data)
```
这里我们使用了Python的with语句来打开文件,这样就不需要手动关闭文件了。read()方法将整个文件读入一个字符串中,并打印出来。
写入文件:
```python
with open('filename.txt', 'w') as file:
file.write('Hello World!')
```
这里我们使用了Python的with语句来打开文件,并指定了文件模式为写入模式。然后我们使用write()方法将字符串写入文件中。
python读取csv数据写入csv
如何用Python读取CSV数据并写入CSV?
要读取CSV文件,可以使用Python标准库中的csv模块。要写入CSV文件,同样可以使用csv模块。
以下是一个简单的例子,读取一个CSV文件并将其写入另一个CSV文件:
```python
import csv
# 读取CSV文件
with open('input.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
data = [row for row in reader]
# 在处理数据时,可以对其进行修改
# 写入CSV文件
with open('output.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerows(data)
```
在上面的代码中,我们首先打开一个输入CSV文件,并使用csv.reader()方法将其读入到一个列表中。然后,我们在对数据进行处理之后,使用csv.writer()方法将数据写入到一个输出CSV文件中。注意要在打开输出文件时使用'w'模式。由于CSV文件中的数据以行为单位,因此在读取和写入文件时需要指定newline参数。
相关推荐
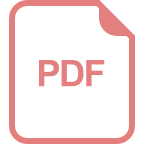
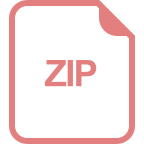
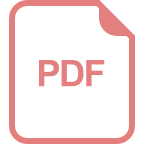












