java编写一个ATM 柜员机模拟程序 模拟 ATM 柜员机的相关功能,实现其存款、取款、余额查询、密码修改等相关功能, 属于验证性实验。目的是通过实验了解图形用户界面程序的开发;图形用户界面设计方法; 常用 swing 组件的使用;事件处理程序设计
时间: 2024-04-30 10:20:46 浏览: 20
好的,以下是一个简单的ATM柜员机模拟程序的Java代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ATM extends JFrame implements ActionListener {
private JTextField tfDeposit;
private JTextField tfWithdraw;
private JLabel lblBalance;
private JPasswordField pfOld;
private JPasswordField pfNew;
private JPasswordField pfConfirm;
private double balance;
private String password;
public ATM() {
super("ATM");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
JLabel lblTitle = new JLabel("ATM");
lblTitle.setFont(new Font("Arial", Font.BOLD, 24));
add(lblTitle, BorderLayout.NORTH);
JPanel panel = new JPanel(new GridLayout(5, 2));
panel.add(new JLabel("Deposit:"));
tfDeposit = new JTextField();
panel.add(tfDeposit);
panel.add(new JLabel("Withdraw:"));
tfWithdraw = new JTextField();
panel.add(tfWithdraw);
panel.add(new JLabel("Balance:"));
lblBalance = new JLabel("0.00");
panel.add(lblBalance);
JButton btnDeposit = new JButton("Deposit");
btnDeposit.addActionListener(this);
panel.add(btnDeposit);
JButton btnWithdraw = new JButton("Withdraw");
btnWithdraw.addActionListener(this);
panel.add(btnWithdraw);
add(panel, BorderLayout.CENTER);
JPanel panel2 = new JPanel(new GridLayout(4, 2));
panel2.add(new JLabel("Old password:"));
pfOld = new JPasswordField();
panel2.add(pfOld);
panel2.add(new JLabel("New password:"));
pfNew = new JPasswordField();
panel2.add(pfNew);
panel2.add(new JLabel("Confirm password:"));
pfConfirm = new JPasswordField();
panel2.add(pfConfirm);
JButton btnChangePassword = new JButton("Change password");
btnChangePassword.addActionListener(this);
panel2.add(btnChangePassword);
add(panel2, BorderLayout.SOUTH);
balance = 1000.00;
password = "1234";
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Deposit")) {
double amount = Double.parseDouble(tfDeposit.getText());
balance += amount;
lblBalance.setText(String.format("%.2f", balance));
tfDeposit.setText("");
} else if (e.getActionCommand().equals("Withdraw")) {
double amount = Double.parseDouble(tfWithdraw.getText());
if (amount > balance) {
JOptionPane.showMessageDialog(this, "Insufficient funds.");
} else {
balance -= amount;
lblBalance.setText(String.format("%.2f", balance));
}
tfWithdraw.setText("");
} else if (e.getActionCommand().equals("Change password")) {
String oldPassword = new String(pfOld.getPassword());
String newPassword = new String(pfNew.getPassword());
String confirmPassword = new String(pfConfirm.getPassword());
if (!oldPassword.equals(password)) {
JOptionPane.showMessageDialog(this, "Incorrect old password.");
} else if (!newPassword.equals(confirmPassword)) {
JOptionPane.showMessageDialog(this, "Passwords do not match.");
} else {
password = newPassword;
JOptionPane.showMessageDialog(this, "Password changed.");
pfOld.setText("");
pfNew.setText("");
pfConfirm.setText("");
}
}
}
public static void main(String[] args) {
ATM atm = new ATM();
atm.setVisible(true);
}
}
```
这个程序使用了Swing组件来创建图形用户界面。它有两个面板,一个面板用于处理存款、取款和余额查询,另一个面板用于处理密码修改。当用户输入存款或取款金额时,程序会更新余额并将其显示在标签上。当用户点击“Change password”按钮时,程序会先检查旧密码是否正确,然后检查新密码是否与确认密码匹配,最后更新密码并显示一条消息框。
相关推荐
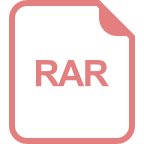
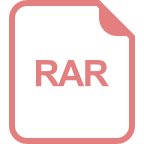
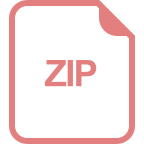














