使用java图形用户界面实现 1)当输出正确的账号和密码的时候,才能登录ATM柜员机系统。功能实现: 1.1)查询余额。初始值自己设置; 1.2)取款,每次取款数额有限,且不能透支; 1.3)存款 1.4)修改密码:新密码不能和旧密码相同。只有旧密码输入正确,才能设置新密码。 给出实现代码
时间: 2024-02-29 09:52:05 浏览: 65
以下是一个简单的实现代码,仅供参考:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ATMSystem extends JFrame {
private String username = "admin"; // 设置默认用户名和密码
private String password = "123456";
private double balance = 10000; // 设置初始余额
private JTextField userField = new JTextField(10);
private JPasswordField pwdField = new JPasswordField(10);
private JButton loginButton = new JButton("登录");
private JButton queryButton = new JButton("查询余额");
private JButton withdrawButton = new JButton("取款");
private JButton depositButton = new JButton("存款");
private JButton changePwdButton = new JButton("修改密码");
public ATMSystem() {
super("ATM柜员机系统");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setLayout(new GridLayout(3, 2));
add(new JLabel("用户名:"));
add(userField);
add(new JLabel("密码:"));
add(pwdField);
add(loginButton);
loginButton.addActionListener(new LoginListener());
queryButton.addActionListener(new QueryListener());
withdrawButton.addActionListener(new WithdrawListener());
depositButton.addActionListener(new DepositListener());
changePwdButton.addActionListener(new ChangePwdListener());
}
private class LoginListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
String usernameInput = userField.getText();
String passwordInput = new String(pwdField.getPassword());
if (usernameInput.equals(username) && passwordInput.equals(password)) { // 检查用户名和密码是否正确
JOptionPane.showMessageDialog(null, "登录成功!");
setVisible(false); // 隐藏登录界面
showMainMenu(); // 显示主菜单
} else {
JOptionPane.showMessageDialog(null, "用户名或密码错误,请重新输入!");
}
}
}
private class QueryListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(null, "当前余额为:" + balance);
}
}
private class WithdrawListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
String input = JOptionPane.showInputDialog(null, "请输入取款金额:");
double amount = Double.parseDouble(input);
if (amount > balance || amount > 1000) { // 检查取款金额是否合法
JOptionPane.showMessageDialog(null, "取款金额不合法!");
} else {
balance -= amount;
JOptionPane.showMessageDialog(null, "取款成功,当前余额为:" + balance);
}
}
}
private class DepositListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
String input = JOptionPane.showInputDialog(null, "请输入存款金额:");
double amount = Double.parseDouble(input);
balance += amount;
JOptionPane.showMessageDialog(null, "存款成功,当前余额为:" + balance);
}
}
private class ChangePwdListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
String oldPwd = JOptionPane.showInputDialog(null, "请输入旧密码:");
if (!oldPwd.equals(password)) { // 检查旧密码是否正确
JOptionPane.showMessageDialog(null, "旧密码错误!");
return;
}
String newPwd = JOptionPane.showInputDialog(null, "请输入新密码:");
if (newPwd.equals(password)) { // 检查新密码是否和旧密码相同
JOptionPane.showMessageDialog(null, "新密码不能和旧密码相同!");
return;
}
password = newPwd; // 更新密码
JOptionPane.showMessageDialog(null, "密码修改成功!");
}
}
private void showMainMenu() {
JFrame mainMenu = new JFrame("ATM主菜单");
mainMenu.setSize(400, 300);
mainMenu.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
mainMenu.setLocationRelativeTo(null);
mainMenu.setLayout(new GridLayout(2, 2));
mainMenu.add(queryButton);
mainMenu.add(withdrawButton);
mainMenu.add(depositButton);
mainMenu.add(changePwdButton);
mainMenu.setVisible(true);
}
public static void main(String[] args) {
new ATMSystem().setVisible(true);
}
}
```
这个代码中包含了登录界面和主菜单界面的设计,以及查询余额、取款、存款、修改密码四个功能的实现。当登录成功后,会隐藏登录界面并显示主菜单界面。每个功能都有对应的监听器实现,点击按钮后会弹出对应的操作界面。注意,这里没有添加密码加密的功能,建议在实际开发中加上。
阅读全文
相关推荐

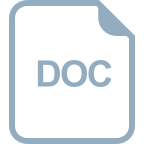
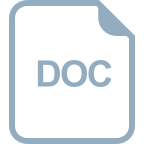
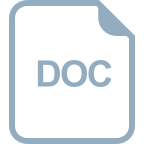
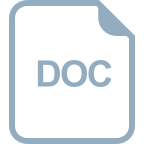
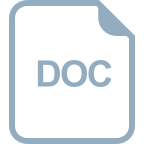
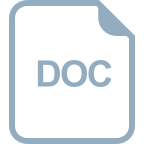
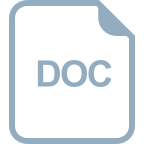
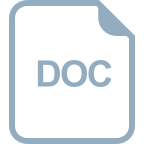
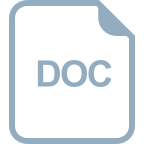
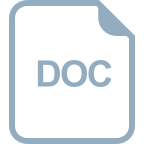


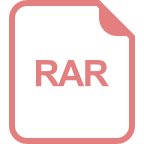
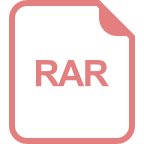