python提取图片中的颜色
时间: 2023-07-30 09:11:47 浏览: 198
可以使用Python的Pillow库来提取图片中的颜色。以下是一个示例代码:
```python
from PIL import Image
import webcolors
# 打开图片
image = Image.open('example.jpg')
# 获取图片中所有像素点的颜色
pixels = image.getcolors(image.size[0] * image.size[1])
# 获取出现最多的颜色
most_frequent_pixel = pixels[0]
for count, color in pixels:
if count > most_frequent_pixel[0]:
most_frequent_pixel = (count, color)
# 将RGB颜色转换为颜色名称
color_name = webcolors.rgb_to_name(most_frequent_pixel[1])
print('The most frequent color in the image is:', color_name)
```
需要注意的是,这个方法只能提取图片中出现最多的颜色,并且只能识别一些常见的颜色名称。如果需要更精确的颜色提取,可以尝试使用一些第三方的色彩分析库。
相关问题
python提取图片中颜色占比
在Python中,你可以使用`PIL`库来处理图像,并结合`sklearn.cluster`模块的KMeans算法来提取主要颜色。这里有一个基本示例说明如何执行这个任务[^1]:
```python
from PIL import Image
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
def extract_colors(image_path, n_colors=5):
# 1. 加载图像并转换为RGB
img = Image.open(image_path)
img_array = np.array(img.convert('RGB'))
# 2. 转换为二维数组并应用KMeans
flat_img = img_array.reshape(-1, 3)
kmeans = KMeans(n_clusters=n_colors)
colors = kmeans.fit_predict(flat_img)
# 3. 计算颜色占比和像素数
color_counts = {color: np.sum(colors == i) for i, color in enumerate(kmeans.cluster_centers_)}
total_pixels = img_array.shape[0] * img_array.shape[1]
# 4. 创建颜色占比和像素统计的数据结构
color_stats = [(k, (v / total_pixels) * 100) for k, v in color_counts.items()]
# 5. 可视化结果
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.pie(color_counts.values(), labels=kmeans.cluster_centers_.astype(str), autopct='%1.1f%%')
plt.title('Color Pie Chart')
plt.subplot(1, 2, 2)
plt.bar(range(n_colors), color_counts.values(), tick_label=[str(i) for i in range(n_colors)])
plt.title('Bar Chart of Color Pixel Counts')
return color_stats
# 示例
image_path = "path_to_your_image.jpg"
colors_and_percentages = extract_colors(image_path)
```
要按照颜色占比对颜色进行排序[^2],可以在最后一步添加:
```python
sorted_color_stats = sorted(color_stats, key=lambda x: x[1], reverse=True)
```
这会返回一个列表,其中的颜色按占比降序排列。
python提取图片像素点颜色
在Python中,我们可以使用PIL(Pillow)库来处理图像并提取像素的颜色。以下是一个简单的示例,展示如何从一张图片中获取每个像素点的颜色:
```python
from PIL import Image
# 打开图片文件
img = Image.open('image.jpg')
# 转换为RGB模式,因为每个像素点都是由红色、绿色和蓝色三个分量组成
rgb_img = img.convert('RGB')
# 获取图片宽度和高度
width, height = rgb_img.size
# 遍历所有像素点
for x in range(width):
for y in range(height):
# 提取当前像素点的RGB值
r, g, b = rgb_img.getpixel((x, y))
# 输出像素点颜色,例如:(255, 0, 0)代表红色
print(f"({r}, {g}, {b})", end=' ')
print() # 每行结束后换行
```
在这个例子中,`getpixel()`函数用于从指定位置(x, y)获取像素点的RGB值。你可以根据需要修改这个脚本来满足特定需求,比如存储到数组或创建一个色彩分布的统计。
阅读全文
相关推荐
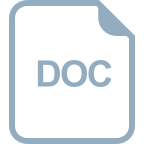
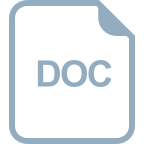
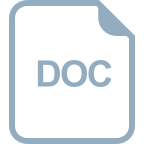
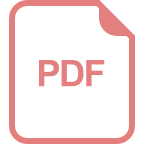












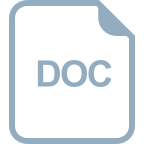