#include <fstream> #include <iostream> using namespace std; int main() { char c; //创建用于格式化输出的文件对象ofs //从键盘读入一行文本,写入文件string.txt中 c = cin.get(); while (c != '\n') { ofs << c; c = cin.get(); } ofs.close(); //创建从string.txt用于格式化输入的文件对象ifs //统计其中的大写字母、小写字母和数字的个数并输出 return 0; }
时间: 2024-02-06 09:04:06 浏览: 84
这段代码是有问题的,因为ofs对象没有被定义和初始化,无法进行文件输出操作。另外,ifs对象也没有被定义和初始化,无法进行文件输入操作。因此,需要加上以下代码进行对象的定义和初始化:
```
ofstream ofs("string.txt"); //定义和初始化输出文件对象
ifstream ifs("string.txt"); //定义和初始化输入文件对象
```
同时,还需要加上代码对大写字母、小写字母和数字进行统计,并输出结果。下面是修正后的代码:
```
#include <fstream>
#include <iostream>
using namespace std;
int main() {
char c;
int upper = 0, lower = 0, digit = 0; //统计大写字母、小写字母和数字的个数
ofstream ofs("string.txt"); //定义和初始化输出文件对象
//从键盘读入一行文本,写入文件string.txt中
c = cin.get();
while (c != '\n') {
ofs << c;
c = cin.get();
}
ofs.close();
ifstream ifs("string.txt"); //定义和初始化输入文件对象
//统计其中的大写字母、小写字母和数字的个数并输出
while (ifs.get(c)) {
if (isupper(c)) {
upper++;
} else if (islower(c)) {
lower++;
} else if (isdigit(c)) {
digit++;
}
}
ifs.close();
cout << "大写字母个数:" << upper << endl;
cout << "小写字母个数:" << lower << endl;
cout << "数字个数:" << digit << endl;
return 0;
}
```
注意,在使用isupper、islower、isdigit这些函数时,需要在程序开头加上头文件<cctype>。
阅读全文
相关推荐

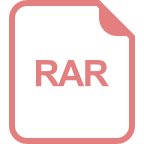


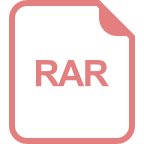













