用python方法设计一个学生类student,包含学生的学号,姓名和python成绩 ,并计算三名学生的平均成绩
时间: 2024-01-03 07:04:29 浏览: 89
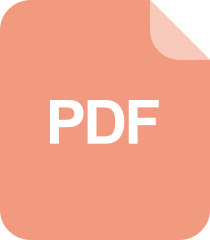
python创建学生成绩管理系统

好的,以下是一个用 Python 实现的学生类 `Student` 的示例代码,并计算三名学生的平均成绩:
```python
class Student:
def __init__(self, id, name, python_score):
self.id = id
self.name = name
self.python_score = python_score
def get_id(self):
return self.id
def get_name(self):
return self.name
def get_python_score(self):
return self.python_score
def set_python_score(self, score):
self.python_score = score
# 创建三个学生对象
s1 = Student(1001, "Tom", 95.5)
s2 = Student(1002, "Jerry", 88.0)
s3 = Student(1003, "Mickey", 79.0)
# 计算三名学生的平均成绩
avg_score = (s1.get_python_score() + s2.get_python_score() + s3.get_python_score()) / 3.0
print("The average score of three students is:", avg_score)
```
在这个示例代码中,我们使用了 Python 中的类来定义了一个名为 `Student` 的学生类,它包含了三个成员变量:`id` 表示学生的学号,`name` 表示学生的姓名,`python_score` 表示学生的 Python 成绩。其中,我们还实现了一些成员方法,包括:
- `__init__(self, id, name, python_score)`:构造函数,用于创建一个学生对象,并初始化其成员变量;
- `get_id(self)`:用于获取学生的学号;
- `get_name(self)`:用于获取学生的姓名;
- `get_python_score(self)`:用于获取学生的 Python 成绩;
- `set_python_score(self, score)`:用于设置学生的 Python 成绩。
在主程序中,我们创建了三个学生对象,并调用它们的成员方法来获取它们的 Python 成绩,然后计算了它们的平均成绩,并输出到控制台上。
阅读全文
相关推荐
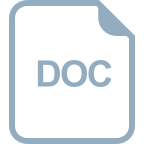
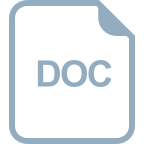















