设计一个学生类student,包含学生的学号,姓名和python成绩 ,并计算三名学生的平均成绩
时间: 2024-01-03 09:03:35 浏览: 89
好的,以下是一个设计学生类 `Student` 的示例代码,并计算三名学生的平均成绩:
```c++
#include <iostream>
#include <string>
class Student {
public:
// 构造函数
Student(int id, std::string name, double python_score)
: id_(id), name_(name), python_score_(python_score) {}
// 获取学生学号
int GetId() const { return id_; }
// 获取学生姓名
std::string GetName() const { return name_; }
// 获取学生 Python 成绩
double GetPythonScore() const { return python_score_; }
// 设置学生 Python 成绩
void SetPythonScore(double score) { python_score_ = score; }
private:
int id_; // 学号
std::string name_; // 姓名
double python_score_; // Python 成绩
};
int main() {
// 创建三个学生对象
Student s1(1001, "Tom", 95.5);
Student s2(1002, "Jerry", 88.0);
Student s3(1003, "Mickey", 79.0);
// 计算三名学生的平均成绩
double avg_score = (s1.GetPythonScore() + s2.GetPythonScore() + s3.GetPythonScore()) / 3.0;
std::cout << "The average score of three students is: " << avg_score << std::endl;
return 0;
}
```
在这个示例代码中,我们使用了 C++ 中的类来定义了一个名为 `Student` 的学生类,它包含了三个成员变量:`id_` 表示学生的学号,`name_` 表示学生的姓名,`python_score_` 表示学生的 Python 成绩。其中,我们还实现了一些成员函数,包括:
- 构造函数:用于创建一个学生对象,并初始化其成员变量;
- `GetId()`:用于获取学生的学号;
- `GetName()`:用于获取学生的姓名;
- `GetPythonScore()`:用于获取学生的 Python 成绩;
- `SetPythonScore()`:用于设置学生的 Python 成绩。
在 `main()` 函数中,我们创建了三个学生对象,并调用它们的成员函数来获取它们的 Python 成绩,然后计算了它们的平均成绩,并输出到控制台上。
阅读全文
相关推荐
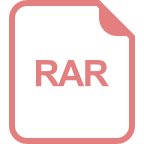















