yolov3人脸检测代码
时间: 2023-07-28 16:06:38 浏览: 62
以下是使用 YOLOv3 进行人脸检测的代码示例。请确保已安装好相关的依赖库和模型文件。
```
import cv2
import numpy as np
# 加载 YOLOv3 模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 加载 COCO 数据集标签
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 设置需要检测的对象为人脸
face_class_id = classes.index("person")
# 加载测试图片
img = cv2.imread("test.jpg")
# 获取图片尺寸
height, width, _ = img.shape
# 将图片转换为 YOLOv3 输入格式
blob = cv2.dnn.blobFromImage(img, 1/255, (416, 416), swapRB=True, crop=False)
# 将输入数据传入模型进行推理
net.setInput(blob)
outs = net.forward(net.getUnconnectedOutLayersNames())
# 解析模型输出结果并进行筛选
conf_threshold = 0.5
nms_threshold = 0.4
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if class_id == face_class_id and confidence > conf_threshold:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = center_x - w // 2
y = center_y - h // 2
boxes.append([x, y, w, h])
# 对检测结果进行非极大值抑制处理
indices = cv2.dnn.NMSBoxes(boxes, [1.0]*len(boxes), conf_threshold, nms_threshold)
for i in indices:
i = i[0]
x, y, w, h = boxes[i]
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示检测结果
cv2.imshow("Face Detection", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,由于 YOLOv3 是基于 COCO 数据集训练的,因此模型输出的类别标签与人脸无关。在上面的代码中,我们通过 `classes.index("person")` 将需要检测的对象设置为人脸。此外,还需要根据实际情况调整 `conf_threshold` 和 `nms_threshold` 参数来控制检测结果的精度和召回率。
相关推荐
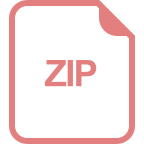
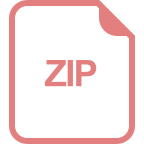
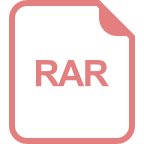
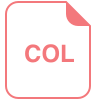
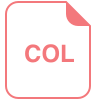
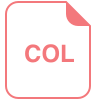
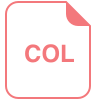
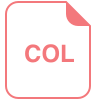









