使用vue根据淘宝写一个商品评论页面代码
时间: 2024-03-11 21:43:38 浏览: 146
好的,下面是使用 Vue 根据淘宝样式编写商品评论页面的代码示例:
1. App.vue
```html
<template>
<div class="app">
<Header />
<CommentList />
<Footer />
</div>
</template>
<script>
import Header from './components/Header.vue'
import CommentList from './components/CommentList.vue'
import Footer from './components/Footer.vue'
export default {
components: {
Header,
CommentList,
Footer
}
}
</script>
```
2. Header.vue
```html
<template>
<div class="header">
<div class="left" @click="goBack">
<i class="iconfont icon-back"></i>
</div>
<div class="title">商品评价</div>
<div class="right"></div>
</div>
</template>
<script>
export default {
methods: {
goBack() {
this.$router.go(-1)
}
}
}
</script>
```
3. CommentList.vue
```html
<template>
<div class="comment-list">
<div class="filter">
<div class="item">全部</div>
<div class="item">好评</div>
<div class="item">中评</div>
<div class="item">差评</div>
</div>
<div class="list">
<div class="item" v-for="(comment, index) in comments" :key="index">
<div class="avatar">
<img :src="comment.avatar" alt="">
</div>
<div class="content">
<div class="nickname">{{ comment.nickname }}</div>
<div class="stars">
<i class="iconfont icon-star"></i>
<i class="iconfont icon-star"></i>
<i class="iconfont icon-star"></i>
<i class="iconfont icon-star"></i>
<i class="iconfont icon-star"></i>
</div>
<div class="text">{{ comment.text }}</div>
<div class="images">
<img v-for="(image, index) in comment.images" :src="image" :key="index" alt="">
</div>
<div class="time">{{ comment.time }}</div>
</div>
</div>
</div>
<div class="load-more" v-if="showLoadMore" @click="loadMore">
点击加载更多
</div>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
comments: [],
page: 1,
pageSize: 10,
showLoadMore: true
}
},
methods: {
loadMore() {
this.page++
this.getComments()
},
getComments() {
axios.get('/api/comments', {
params: {
page: this.page,
pageSize: this.pageSize
}
}).then(res => {
const data = res.data
if (data.length) {
this.comments = [...this.comments, ...data]
} else {
this.showLoadMore = false
}
}).catch(err => {
console.log(err)
})
}
},
mounted() {
this.getComments()
}
}
</script>
```
4. Footer.vue
```html
<template>
<div class="footer">
<form class="form" @submit.prevent="submitComment">
<textarea class="textarea" v-model="comment" placeholder="请输入评论内容"></textarea>
<div class="btn-wrapper">
<button class="btn">提交评论</button>
</div>
</form>
</div>
</template>
<script>
export default {
data() {
return {
comment: ''
}
},
methods: {
submitComment() {
if (!this.comment.trim()) {
return
}
// 发送评论数据到后台
// ...
// 清空评论框内容
this.comment = ''
}
}
}
</script>
```
以上就是使用 Vue 根据淘宝样式编写商品评论页面的代码示例,希望对你有所帮助。
阅读全文
相关推荐
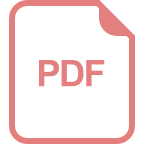
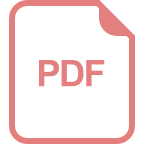
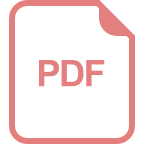
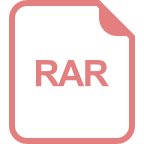
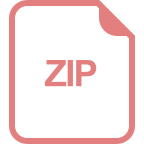
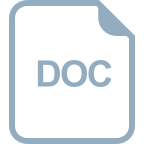









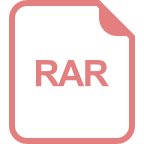
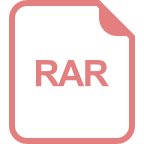
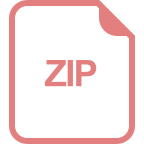