java Stream流数组对象根据某个属性去重方法
时间: 2023-09-26 13:11:31 浏览: 125
可以通过使用Java 8的Stream流和Collectors工具类来根据某个属性去重数组对象。
假设有一个名为Person的类,其中有两个属性name和age,我们想根据name属性去重Person对象数组。
可以使用Stream的distinct()方法和Collectors.toMap()方法来实现:
```
Person[] persons = {new Person("John", 20), new Person("Tom", 25), new Person("John", 30)};
Map<String, Person> map = Arrays.stream(persons)
.distinct()
.collect(Collectors.toMap(Person::getName, Function.identity()));
Person[] result = map.values().toArray(new Person[map.size()]);
```
首先,通过Arrays.stream()方法将Person对象数组转换为Stream流。
然后,使用distinct()方法去重,该方法默认使用对象的equals()方法进行比较。
最后,使用Collectors.toMap()方法将Stream流转换为Map集合,key为Person对象的name属性,value为Person对象本身。
最终,通过Map的values()方法获取不重复的Person对象集合,并将其转换为数组返回。
相关问题
list对象数组根据某个属性去重java
你可以使用Java 8中的Stream API来根据某个属性对list对象数组进行去重。具体实现如下:
假设你有一个包含Person对象的List:
```
List<Person> people = Arrays.asList(
new Person("John", 25),
new Person("Bob", 30),
new Person("John", 25),
new Person("Alice", 20)
);
```
如果你想根据Person对象的name属性去重,可以使用以下代码:
```
List<Person> distinctPeople = people.stream()
.filter(distinctByKey(Person::getName))
.collect(Collectors.toList());
```
其中,`distinctByKey`是一个自定义的方法,用于根据某个属性去重:
```
public static <T> Predicate<T> distinctByKey(Function<? super T, ?> keyExtractor) {
Map<Object, Boolean> seen = new ConcurrentHashMap<>();
return t -> seen.putIfAbsent(keyExtractor.apply(t), Boolean.TRUE) == null;
}
```
上述代码会返回一个新的List,其中包含去重后的Person对象:
```
[Person(name=John, age=25), Person(name=Bob, age=30), Person(name=Alice, age=20)]
```
注意:该方法仅适用于Java 8及以上版本。如果你使用的是早期版本的Java,你可以使用传统的方式来实现去重,例如使用HashSet等数据结构来去重。
java Stream流数组去重方法
Java 8引入了Stream API,使得对集合和数组的处理更加简洁、高效。在Stream中,可以使用distinct()方法来对元素进行去重。
对于数组,可以先将其转换成Stream,再使用distinct()方法进行去重。示例代码如下:
```
int[] arr = {1, 2, 3, 2, 4, 5, 3, 6};
int[] arrDistinct = Arrays.stream(arr).distinct().toArray();
```
以上代码将数组arr转换成Stream,使用distinct()方法进行去重,再将结果转换成数组arrDistinct。最终得到的arrDistinct为{1, 2, 3, 4, 5, 6},去重后的结果。
需要注意的是,数组中的元素必须实现equals()方法和hashCode()方法,才能进行去重。否则,distinct()方法无法正确判断元素是否重复。如果数组中的元素是自定义对象,需要自行实现equals()方法和hashCode()方法。
阅读全文
相关推荐
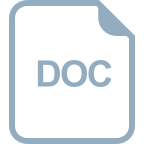
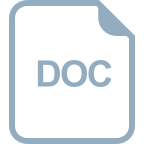
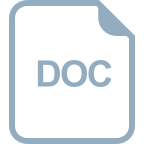










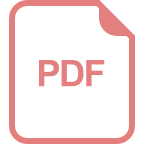


