next_permutation怎么用
时间: 2023-05-27 14:07:50 浏览: 69
next_permutation是C++ STL中的一个函数,用于将一个排列转换为下一个排列。具体用法如下:
1. 首先需要包含头文件<algorithm>
2. 将要排列的序列存入一个vector中
3. 使用next_permutation函数对vector进行排列
示例代码如下:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main()
{
vector<int> v = {1, 2, 3};
do {
for (int i : v) {
cout << i << " ";
}
cout << endl;
} while (next_permutation(v.begin(), v.end()));
return 0;
}
```
这段代码中,我们定义了一个vector v,包含了三个元素1、2、3。使用do-while循环以及next_permutation函数对v进行排列,并将排列后的结果输出。输出结果如下:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
可以看到,next_permutation函数将一个排列转换为下一个排列。如果下一个排列不存在,函数将返回false,否则返回true。因此,我们可以使用do-while循环来遍历所有的排列。
相关问题
next_permutation使用方法
next_permutation() 是一个 C++ STL 函数,它可以用于生成下一个排列。
使用方法:
1. 首先需要包含头文件 `#include<algorithm>`
2. 然后定义一个存储排列的数组或容器,比如 `vector<int> v`,并对其进行初始化。
3. 调用 next_permutation() 函数,该函数的参数是一个迭代器范围,表示排列的起始和终止位置。例如:
```c++
next_permutation(v.begin(), v.end());
```
4. 如果当前排列的下一个排列存在,则函数会返回 true,并将当前排列修改成下一个排列。如果当前排列已经是最后一个排列,则函数会返回 false,并将当前排列修改成第一个排列。
下面是一个示例程序,演示了如何使用 next_permutation() 函数:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3};
do {
for (int i : v) {
cout << i << " ";
}
cout << endl;
} while (next_permutation(v.begin(), v.end()));
return 0;
}
```
输出结果为:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
该程序首先定义了一个包含三个整数的 vector v,然后使用 do-while 循环不断生成下一个排列,并输出每个排列。最终输出了所有的 3! = 6 种排列。
next_permutation
The `next_permutation()` function is a C++ standard library function that generates the next lexicographically greater permutation of a sequence. In other words, it rearranges the elements of a sequence in such a way that the lexicographically next higher permutation is obtained. If the current sequence is already the highest possible permutation, it rearranges it to the lowest possible permutation.
Here is the syntax for using `next_permutation()` function:
```
template<class BidirectionalIterator>
bool next_permutation(BidirectionalIterator first, BidirectionalIterator last);
```
The function takes two iterators, `first` and `last`, which define the range of the sequence to be modified. The function returns `true` if a lexicographically greater permutation exists, and `false` otherwise. If the function returns `true`, the sequence is modified to the next permutation.
Here is an example of using the `next_permutation()` function:
```
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3};
do {
for (int x : v) {
std::cout << x << ' ';
}
std::cout << '\n';
} while (std::next_permutation(v.begin(), v.end()));
return 0;
}
```
This code generates all possible permutations of the vector `{1, 2, 3}` and prints them to the console.
阅读全文
相关推荐
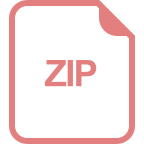
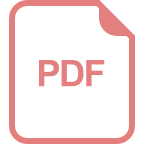
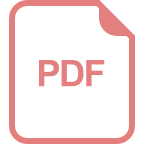











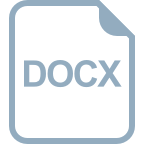