pyqt5 父类窗口class Ui_MainWindow()有一个控件QGraphicsView,怎么样让子类QGraphicsView继承父类Ui_MainWindow,可以进行图片显示,滑轮滚动放大缩小
时间: 2024-02-02 07:42:54 浏览: 141
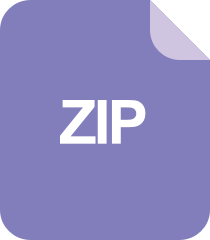
PYQT5、PYQT5-Tools离线安装包
可以通过以下步骤实现:
1. 在子类中继承父类,即在子类的类定义中添加父类的名称,如下所示:
```python
class MyGraphicsView(Ui_MainWindow, QGraphicsView):
def __init__(self):
super(MyGraphicsView, self).__init__()
```
2. 在子类的 `__init__` 方法中,调用父类的 `setupUi` 方法来初始化UI组件,如下所示:
```python
class MyGraphicsView(Ui_MainWindow, QGraphicsView):
def __init__(self):
super(MyGraphicsView, self).__init__()
self.setupUi(self)
```
3. 在 `QGraphicsView` 控件中加载图片,并设置滚轮事件,如下所示:
```python
class MyGraphicsView(Ui_MainWindow, QGraphicsView):
def __init__(self):
super(MyGraphicsView, self).__init__()
self.setupUi(self)
# 加载图片
self.scene = QGraphicsScene()
self.image = QImage("image.jpg")
self.scene.addPixmap(QPixmap.fromImage(self.image))
self.setScene(self.scene)
# 设置滚轮事件
self.setRenderHint(QPainter.Antialiasing)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setVerticalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setHorizontalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setTransformationAnchor(QGraphicsView.AnchorUnderMouse)
self.setResizeAnchor(QGraphicsView.AnchorUnderMouse)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setInteractive(True)
self.wheelEvent = self.zoom
```
4. 实现滚轮事件中的放大缩小逻辑,如下所示:
```python
class MyGraphicsView(Ui_MainWindow, QGraphicsView):
def __init__(self):
super(MyGraphicsView, self).__init__()
self.setupUi(self)
# 加载图片
self.scene = QGraphicsScene()
self.image = QImage("image.jpg")
self.scene.addPixmap(QPixmap.fromImage(self.image))
self.setScene(self.scene)
# 设置滚轮事件
self.setRenderHint(QPainter.Antialiasing)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setVerticalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setHorizontalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setTransformationAnchor(QGraphicsView.AnchorUnderMouse)
self.setResizeAnchor(QGraphicsView.AnchorUnderMouse)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setInteractive(True)
self.wheelEvent = self.zoom
# 实现滚轮事件中的放大缩小逻辑
def zoom(self, event):
if event.angleDelta().y() > 0:
self.scale(1.1, 1.1)
else:
self.scale(1 / 1.1, 1 / 1.1)
```
通过以上步骤,就可以实现子类继承父类并且在 `QGraphicsView` 控件中显示图片,并且支持滚轮放大缩小功能。
阅读全文
相关推荐
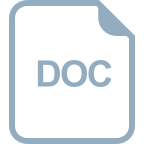
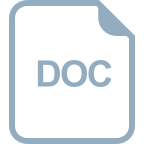

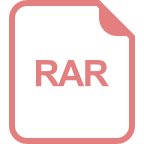
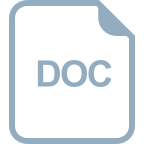












