linux使用golang实现tun虚拟网卡读写
时间: 2023-11-02 10:11:18 浏览: 65
以下是一个简单的示例代码,使用golang实现tun虚拟网卡的读写:
```go
package main
import (
"fmt"
"net"
"os"
"syscall"
)
const (
MTU = 1500
IFNAME = "tun0"
IFPREFIX = "/dev/net/tun"
)
func main() {
// 打开tun设备
fd, err := syscall.Open(IFPREFIX, os.O_RDWR, 0)
if err != nil {
fmt.Println("Open tun device failed:", err)
return
}
defer syscall.Close(fd)
// 设置tun设备
ifr := &syscall.Ifreq{}
copy(ifr.Name[:], IFNAME)
ifr.Flags = syscall.IFF_TUN | syscall.IFF_NO_PI
_, _, err = syscall.Syscall(syscall.SYS_IOCTL, uintptr(fd), syscall.TUNSETIFF, uintptr(unsafe.Pointer(ifr)))
if err != 0 {
fmt.Println("Set tun device failed:", err)
return
}
// 获取tun设备的IP地址和子网掩码
iface, err := net.InterfaceByName(IFNAME)
if err != nil {
fmt.Println("Get interface failed:", err)
return
}
addrs, err := iface.Addrs()
if err != nil {
fmt.Println("Get address failed:", err)
return
}
for _, addr := range addrs {
ip, _, err := net.ParseCIDR(addr.String())
if err == nil && ip.To4() != nil {
fmt.Println("IP Address:", ip)
fmt.Println("Subnet Mask:", net.IPv4Mask(255, 255, 255, 0))
break
}
}
// 读取数据
buf := make([]byte, MTU)
for {
n, err := syscall.Read(fd, buf)
if err != nil {
fmt.Println("Read from tun device failed:", err)
return
}
fmt.Println("Read:", buf[:n])
}
// 写入数据
data := []byte("Hello, world!")
n, err := syscall.Write(fd, data)
if err != nil {
fmt.Println("Write to tun device failed:", err)
return
}
fmt.Println("Write:", n)
}
```
该代码首先打开tun设备,并设置为tun模式。然后获取tun设备的IP地址和子网掩码,并开始读取数据。在读取到数据后,将数据打印出来。最后,该代码将Hello, world!写入tun设备。
需要注意的是,该代码需要root权限才能运行。
相关推荐
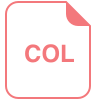
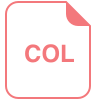












