@RequestMapping(value = "notices", method = RequestMethod.GET) public PageInfo<Notice> list(@RequestParam(value = "start", defaultValue = "1") int start, @RequestParam(value = "size", defaultValue = "10") int size, @RequestParam(value = "keyword", defaultValue = "") String keyword) { PageHelper.startPage(start, size, "createtime desc"); Map<String, Object> paramMap = new HashMap<String, Object>(); if (!StringUtils.isEmpty(keyword)) { paramMap.put("keyword", keyword); } List<Notice> list = noticeService.list(paramMap); PageInfo<Notice> page = new PageInfo<Notice>(list, 5); return page; }
时间: 2024-03-04 15:53:28 浏览: 36
这段代码是一个基于 Spring MVC 的控制器方法,用于处理 GET 请求,访问路径为 "/notices"。它通过 @RequestParam 注解获取请求参数,并调用 noticeService 中的 list 方法查询符合条件的公告列表,并使用 PageHelper 分页插件进行分页。最后,将查询结果封装为 PageInfo 对象并返回给客户端。
具体解释如下:
1. @RequestMapping:用于映射请求路径和 HTTP 请求方法。
2. @RequestParam:用于获取请求参数。
3. start、size、keyword:请求参数,其中 start 表示当前页码,size 表示每页显示的记录数,keyword 表示查询关键字。
4. PageHelper.startPage:使用 PageHelper 分页插件进行分页,其中 start 表示当前页码,size 表示每页显示的记录数,"createtime desc" 表示按创建时间倒序排序。
5. paramMap:存储查询参数的 Map。
6. StringUtils.isEmpty:判断字符串是否为空。
7. noticeService.list:调用 NoticeService 中的 list 方法进行查询,返回一个 Notice 类型的 List。
8. PageInfo:将查询结果封装为一个 PageInfo 对象,其中 list 表示查询结果,5 表示分页导航条显示的页码数量。
9. 返回 PageInfo 对象。
相关问题
@RequestMapping(method = RequestMethod.POST)和@RequestMapping(method = RequestMethod.GET)的区别
@RequestMapping(method = RequestMethod.POST)和@RequestMapping(method = RequestMethod.GET)的区别在于它们用于指定不同的HTTP请求方法。
1. @RequestMapping(method = RequestMethod.POST):这个注解用于指定处理POST请求的方法。当客户端发送一个POST请求时,服务器将调用带有该注解的方法来处理请求。
2. @RequestMapping(method = RequestMethod.GET):这个注解用于指定处理GET请求的方法。当客户端发送一个GET请求时,服务器将调用带有该注解的方法来处理请求。
这两个注解的区别在于它们指定的HTTP请求方法不同,一个是POST,一个是GET。根据不同的请求方法,服务器将调用相应的方法来处理请求。
范例:
```java
@RequestMapping(value = "/orders", method = RequestMethod.POST)
public void createOrder() {
// 处理POST请求的逻辑
}
@RequestMapping(value = "/orders", method = RequestMethod.GET)
public void getOrder() {
// 处理GET请求的逻辑
}
```
@RequestMapping(value="/",method=RequestMethod.GET)
`@RequestMapping(value="/",method=RequestMethod.GET)` 是一个用于定义请求映射的注解。它指定了一个URL路径为"/",请求方法为GET的处理方法。
以下是一个使用`@RequestMapping`注解的示例:
```java
@RequestMapping(value="/", method=RequestMethod.GET)
public String home() {
return "Welcome to the home page!";
}
```
这个示例中,当用户发送GET请求到根路径"/"时,将会调用`home()`方法,并返回"Welcome to the home page!"字符串作为响应。
相关推荐
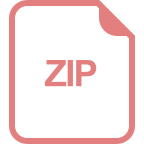
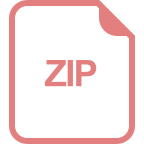
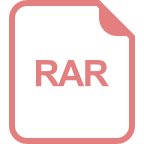












