package com.ssm.controller; import com.ssm.pojo.Record; import com.ssm.service.RecordService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.format.annotation.DateTimeFormat; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import java.util.Date; import java.util.List; @Controller public class recordController { @Autowired private RecordService recordService; @RequestMapping(value = "/toAllRecord",method = RequestMethod.GET) public String toAllRecord(){ return "allrecord"; } @RequestMapping(value = "/allrecord",method = RequestMethod.GET) public String getAllRecord(Model model){ List<Record> transactions = recordService.selectAll(); System.out.println(transactions); model.addAttribute("transactions", transactions); return "allrecord"; } @GetMapping("/recordByNum") public String getRecordByCardNum(@RequestParam("accountNumber") String accountNumber,Model model) { List<Record> transactions = recordService.selectRecordByAccountNumber(accountNumber); System.out.println(transactions); model.addAttribute("transactions", transactions); return "recordByNum"; } @RequestMapping(value = "/toRecordByNum",method = RequestMethod.GET) public String toRecordByNum(){ return "recordByNum"; } @RequestMapping(value = "/toIndex",method = RequestMethod.GET) public String toIndex(){ return "index"; } }
时间: 2024-03-18 22:45:33 浏览: 115
这是一个基于Spring框架的Java web应用的Controller类,用于处理与账户交易记录相关的HTTP请求。其中包括以下方法:
1. toAllRecord():处理访问“/toAllRecord”路径的GET请求,返回字符串“allrecord”,用于跳转到账户交易记录页面。
2. getAllRecord():处理访问“/allrecord”路径的GET请求,调用RecordService服务层的selectAll()方法获取所有的交易记录,将其存储在Model对象中,返回字符串“allrecord”,用于展示所有的账户交易记录。
3. getRecordByCardNum():处理访问“/recordByNum”路径的GET请求,通过@RequestParam注解获取请求中的accountNumber参数,调用RecordService服务层的selectRecordByAccountNumber()方法获取指定账户的交易记录,将其存储在Model对象中,返回字符串“recordByNum”,用于展示指定账户的所有交易记录。
4. toRecordByNum():处理访问“/toRecordByNum”路径的GET请求,返回字符串“recordByNum”,用于跳转到指定账户的交易记录页面。
5. toIndex():处理访问“/toIndex”路径的GET请求,返回字符串“index”,用于跳转到网站首页。
相关问题
There is no getter for property named 'eamil' in 'class com.bdqn.ssm.pojo.Employee'
根据提供的引用内容,可以看出在类`com.bdqn.ssm.pojo.Employee`中没有名为`email`的属性的getter方法。这意味着在该类中无法直接获取`email`属性的值。
如果需要获取`email`属性的值,可以考虑以下几种方法:
1. 检查类定义:首先,确保在`com.bdqn.ssm.pojo.Employee`类的定义中是否存在`email`属性。如果不存在,可以考虑在类中添加该属性,并为其提供相应的getter方法。
2. 使用反射:如果无法修改类定义,可以尝试使用Java的反射机制来获取`email`属性的值。通过使用`Class`类的`getMethod`方法和`invoke`方法,可以动态调用类中的方法,包括获取属性的值。
3. 使用其他方式获取属性值:如果以上方法都不可行,可以考虑使用其他方式获取`email`属性的值。例如,可以通过调用类中其他方法或访问相关的数据库或文件来获取属性的值。
请注意,以上方法仅为一般建议,具体的解决方案取决于具体的代码和需求。
<bean class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="configLocation" value="classpath:mybatis-config.xml"/> <property name="dataSource" ref="dataSource"/> <property name="typeAliasesPackage" value="com.hu.ssm.pojo"/> </bean>
这是一个 Spring 配置文件中配置 MyBatis 的 SqlSessionFactoryBean 的代码片段。其中,configLocation 属性指定了 MyBatis 的配置文件路径,dataSource 属性指定了数据源,typeAliasesPackage 属性指定了 POJO 类的包路径。这个 Bean 的作用是创建 SqlSessionFactory 实例,用于管理 MyBatis 的 SqlSession。
阅读全文
相关推荐
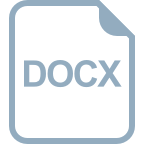
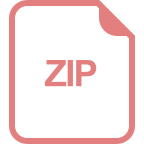
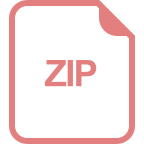













