python 自带解密
时间: 2023-09-11 12:13:26 浏览: 125
Python自带的库中没有专门用于解密的模块,但可以使用一些其他的库来实现解密功能。例如,可以使用`cryptography`库来进行加密和解密操作。以下是一个示例代码:
```python
from cryptography.fernet import Fernet
def decrypt_message(key, ciphertext):
f = Fernet(key)
decrypted_message = f.decrypt(ciphertext)
return decrypted_message.decode()
# 假设已知密钥和密文
key = b'your_encryption_key_here'
ciphertext = b'your_encrypted_message_here'
decrypted_message = decrypt_message(key, ciphertext)
print(decrypted_message)
```
请注意,为了解密一个消息,你需要知道正确的密钥。此外,具体的解密方法取决于你使用的加密算法和密钥管理方式。
相关问题
使用python自带模块,简单加密文件
可以使用 Python 标准库中的 base64 模块对文件进行简单加密。
首先,将文件读入内存中:
```python
with open('filename', 'rb') as f:
content = f.read()
```
然后,使用 base64 编码将内容转换为字符串:
```python
import base64
encoded = base64.b64encode(content)
```
将编码后的字符串写入文件:
```python
with open('encoded_filename', 'wb') as f:
f.write(encoded)
```
解密时,先将编码后的字符串读入内存中:
```python
with open('encoded_filename', 'rb') as f:
encoded_content = f.read()
```
然后,使用 base64 解码将字符串转换回原始内容:
```python
decoded = base64.b64decode(encoded_content)
```
将解码后的内容写入文件:
```python
with open('decoded_filename', 'wb') as f:
f.write(decoded)
```
这样就完成了简单的加密和解密过程。需要注意的是,这种加密方式并不是非常安全,只能防止一些简单的窥探,更高级的加密方式需要使用专业的加密算法。
使用python自带模块,用字符串密码加密文件
你可以使用 Python 标准库中的 `cryptography` 模块来进行加密。
下面是一个示例代码,用于使用密码加密文件:
```python
from cryptography.fernet import Fernet
# 生成一个随机的密钥
key = Fernet.generate_key()
# 将密钥保存到文件中
with open("key.key", "wb") as key_file:
key_file.write(key)
# 读取要加密的文件
with open("plaintext.txt", "rb") as plaintext_file:
plaintext = plaintext_file.read()
# 使用密钥创建一个加密器对象
cipher = Fernet(key)
# 加密文件内容
encrypted = cipher.encrypt(plaintext)
# 将加密后的内容保存到文件中
with open("encrypted.bin", "wb") as encrypted_file:
encrypted_file.write(encrypted)
```
这段代码将会生成一个随机的密钥,将其保存到文件中。然后读取要加密的文件,使用密钥创建加密器对象,加密文件内容并将其保存到文件中。
如果需要解密文件,则可以使用相同的密钥和加密器对象来解密文件。
阅读全文
相关推荐
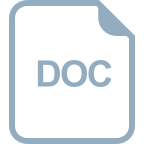
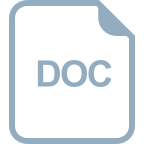
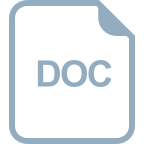
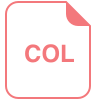
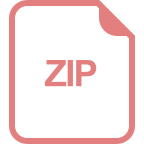
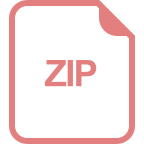
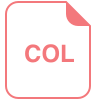









