Course Exercise 1 Restore the elements in an array reversely. E.g.8,6,5,4,1->1,4,5,6,8
时间: 2024-10-21 22:03:25 浏览: 16
Sure! Course Exercise 1 seems to be asking you to reverse the order of elements in an array. Here's a simple C function that demonstrates how to achieve this:
```c
#include <stdio.h>
// Function to reverse an array
void reverse_array(int arr[], int size) {
int start = 0;
int end = size - 1;
// Loop until start is less than end
while (start < end) {
// Swap the elements at start and end indices
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
// Move towards the center by incrementing start and decrementing end
start++;
end--;
}
}
int main() {
int numbers[] = {8, 6, 5, 4, 1};
int size = sizeof(numbers) / sizeof(numbers[0]);
printf("Original array: ");
for (int i = 0; i < size; i++) {
printf("%d ", numbers[i]);
}
// Call the function to reverse the array
reverse_array(numbers, size);
printf("\nReversed array: ");
for (int i = 0; i < size; i++) {
printf("%d ", numbers[i]);
}
return 0;
}
```
This code defines a `reverse_array` function that takes an integer array and its size as parameters. It iterates over the array from both ends and swaps the elements until it reaches the middle. After calling the `reverse_array`, the main function prints the original and reversed arrays.
To run the program or understand the concept, try compiling and executing this code on your local machine, or modify it according to your needs.
阅读全文
相关推荐
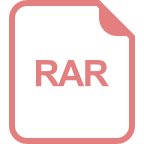
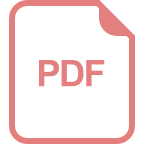
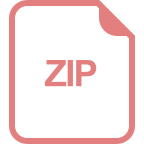
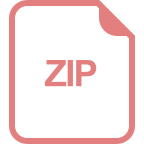
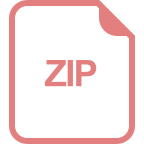
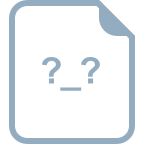
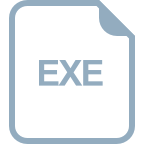
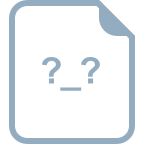
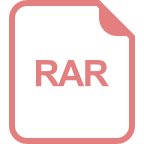
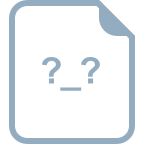
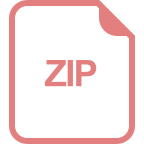
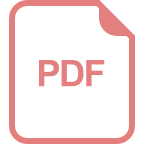
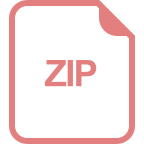
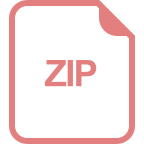
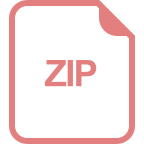