python一个线程,如何获取另一个线程里的变量
时间: 2024-09-27 08:11:40 浏览: 38
在Python中,由于全局解释器锁(GIL)的存在,多线程并不能像多进程那样并行地访问和修改共享数据,因为同一时间只有一个线程在执行。但是,如果需要在多个线程间共享数据,可以采用以下几种方法:
1. **共享数据结构(如list、dict等)**:将变量作为列表或字典的一部分,并确保所有线程都有权限读写这个数据结构。不过这可能导致竞态条件,除非有适当的同步措施。
```python
import threading
shared_variable = [0]
def thread_func():
global shared_variable
# 对共享变量进行操作...
thread_1 = threading.Thread(target=thread_func)
thread_2 = threading.Thread(target=thread_func)
thread_1.start()
thread_2.start()
thread_1.join()
thread_2.join()
```
2. **队列(Queue)**:使用`queue`模块创建线程安全的消息队列,可以在两个线程之间传递数据。
```python
from queue import Queue
queue = Queue()
...
queue.put(some_value)
result = queue.get()
```
3. **线程局部存储(Thread Local Storage, TLS)**:通过`threading.local()`创建线程本地存储,每个线程有自己的独立副本。
```python
import threading
class ThreadLocalStorage:
def __init__(self):
self.value = 0
local_storage = ThreadLocalStorage()
def thread_func():
local_storage.value += 1
thread_1 = threading.Thread(target=thread_func)
thread_2 = threading.Thread(target=thread_func)
thread_1.start()
thread_2.start()
```
4. **锁(Locks)**:如果你确实需要在多线程环境下直接操作某个变量,使用`threading.Lock()`来保护它。但这通常会限制并发性能。
```python
import threading
variable = 0
lock = threading.Lock()
def thread_func():
with lock:
variable += 1
# ...
```
阅读全文
相关推荐
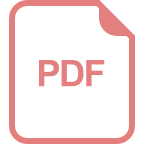
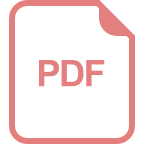
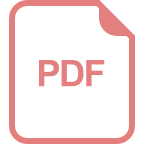















