python jsonl读取
时间: 2023-10-18 09:58:31 浏览: 265
你可以使用 Python 的 `jsonlines` 库来读取 JSONL 文件。JSONL 文件是种每行都是一个独立的 JSON 对象的文件格式。下面是一个简单的示例代码:
```python
import jsonlines
# 打开 JSONL 文件
with jsonlines.open('data.jsonl') as reader:
# 逐行读取 JSON 对象
for obj in reader:
# 处理每个 JSON 对象
print(obj)
```
在上面的代码中,我们使用 `jsonlines.open()` 函数打开 JSONL 文件。然后,我们可以使用 `for` 循环逐行读取 JSON 对象,并在循环中处理每个对象。你可以根据需要修改代码以适应你的具体情况。
请确保在运行代码之前安装了 `jsonlines` 库。你可以使用以下命令来安装它:
```
pip install jsonlines
```
希望对你有帮助!如果有任何进一步的问题,请随时提问。
相关问题
python jsonl读写
在Python中,JSONL(JSON Lines)是一种将JSON数据存储为行的形式,每一行对应一个独立的JSON对象。这种格式便于处理大量数据,因为它们可以方便地逐行读取和写入,而不必将整个文件加载到内存中。
**读取JSONL文件:**
使用`json`模块的`load`方法配合文件迭代器`open`,可以逐行读取JSONL文件中的内容:
```python
import json
def read_jsonl(file_path):
with open(file_path, 'r') as file:
for line in file:
json_data = json.loads(line)
# 处理每个json对象
process_json(json_data)
# 示例调用
read_jsonl('data.jsonl')
```
**写入JSONL文件:**
`json.dump`方法也可以用于逐行写入,只需要将每条数据包裹在`json.dumps`后的列表里:
```python
def write_jsonl(file_path, json_list):
with open(file_path, 'w') as file:
for data in json_list:
file.write(json.dumps(data) + '\n')
# 示例调用
data = [{"name": "Alice", "age": 30}, {"name": "Bob", "age": 35}]
write_jsonl('output.jsonl', data)
```
**相关问题--:**
1. JSONL格式的优点是什么?
2. 如果数据文件非常大,如何更有效地处理?
3. Python中有没有现成的库可以简化JSONL的读写操作?
python读取jsonl文件
你可以使用Python内置的json库来读取jsonl文件。这里提供一个示例代码:
```python
import json
with open('file.jsonl', 'r') as f:
for line in f:
data = json.loads(line)
# 处理data数据
```
在这个示例中,我们打开一个名为“file.jsonl”的jsonl文件,并使用for循环逐行读取文件内容。我们使用json.loads()方法将每行的JSON字符串转换为Python对象。接下来,您可以对数据进行必要的处理。
阅读全文
相关推荐
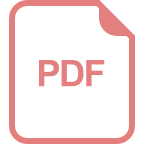
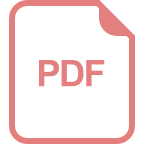
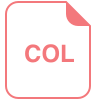











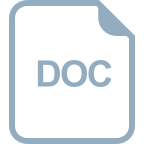