prev_char, current_char = '', ''
时间: 2024-10-29 18:18:56 浏览: 22
在Python中,当你看到这样的变量初始化 `prev_char, current_char = '',```,这意味着你在创建两个名为`prev_char`和`current_char`的变量,并分别将它们的初始值设置为空字符串('',)。空字符串是一个只包含一个空格的字符序列,其长度为0。
这两个变量一般用于跟踪输入流(如用户输入、文件读取等)中的字符,`prev_char`存储的是前一个字符,`current_char`存储当前正在处理的字符。比如在循环中逐字符分析字符串时,可以先检查前一个字符对当前字符的影响,然后更新这两个变量的值。
举个例子:
```python
input_string = "Hello, World!"
i = 0
while i < len(input_string):
prev_char = current_char
current_char = input_string[i]
# 这里可以添加一些基于 prev_char 和 current_char 的操作
print(f"Current character: {current_char}, Previous character: {prev_char}")
i += 1
```
相关问题
写一个这样的代码void insert_item(char *, float, float) -add a new item and its price into linked list
Sure, here's an example implementation of the `insert_item` function that adds a new item and its price into a linked list:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Node {
char *name;
float price;
float amount_paid;
struct Node *next;
} Node;
Node *head = NULL;
void insert_item(char *name, float price, float amount_paid) {
Node *new_node = (Node*) malloc(sizeof(Node));
new_node->name = strdup(name);
new_node->price = price;
new_node->amount_paid = amount_paid;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
} else {
Node *current = head;
Node *prev = NULL;
while (current != NULL && current->price > price) {
prev = current;
current = current->next;
}
if (prev == NULL) {
new_node->next = head;
head = new_node;
} else {
prev->next = new_node;
new_node->next = current;
}
}
}
```
This function creates a new node with the provided name, price, and amount_paid values, and then inserts it into the linked list in descending order based on the price. If the list is empty, the new node is set as the head. If not, the function iterates through the list until it finds the correct position to insert the new node, and adjusts the pointers accordingly.
Note that this implementation assumes that the linked list is already defined and initialized elsewhere in the program.
typedef struct heap info { mstate ar ptr; structheap info *prev; /* Arena for this heap. */ /* Previous heap. */ size t size; /* Current size in bytes. */ /* Size in bytes that has been mprotectedsize t mprotect size;PROT READIPROT WRITE .* Make sure the following data is properly aligned,particularlythat sizeof (heap info) + 2 * SIZE SZ is a multiple of MALLOC ALIGNMENT,*/char pad[-6 * SIZE SZ & MALLOC ALIGN MASKI;}heap info
这段代码定义了一个名为 `heap info` 的结构体类型,包含以下成员变量:
- `mstate ar ptr`:指向 `mstate` 类型的指针变量,表示该堆所属的内存分配状态。
- `struct heap info *prev`:指向前一个堆的指针变量。
- `size t size`:表示当前堆的大小(以字节为单位)。
- `size t mprotect size`:表示已经被 mprotect(内存保护函数)保护的大小(以字节为单位)。
- `char pad[-6 * SIZE SZ & MALLOC ALIGN MASK]`:用于填充使得 `sizeof (heap info) + 2 * SIZE SZ` 能够按照所需的内存对齐方式对齐。
这段代码可能是某个内存管理系统或者堆分配器的一部分,具体实现可能还涉及其他函数和数据结构。
阅读全文
相关推荐














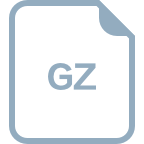

