基于tensorflow生成一段读入图片并修改像素后保存图片的代码
时间: 2024-09-22 12:05:00 浏览: 32
在TensorFlow中,可以使用`tf.image`模块对图片进行处理,包括读取、修改像素值以及保存。下面是一个基本的例子,它会读取一张图片,随机改变一部分像素的颜色,然后保存新的图片:
```python
import tensorflow as tf
# 读取图片
def read_and_decode_image(file_path):
img = tf.io.read_file(file_path)
img = tf.image.decode_jpeg(img, channels=3)
return img
# 修改像素值 (这里使用随机色彩扰动作为示例)
def modify_pixels(image):
image = tf.cast(image, tf.float32) / 255.0 # 将图片归一化到0-1之间
num_pixels_to_change = int(0.1 * tf.reduce_prod(tf.shape(image))) # 变换10%的像素
mask = tf.random.uniform(shape=tf.shape(image)[:2], minval=0, maxval=1, dtype=tf.bool) # 随机选择像素
new_values = tf.where(mask, tf.random.uniform(tf.shape(mask), 0, 256, tf.int32), image) # 改变选中的像素
modified_image = tf.where(mask, new_values, image) # 用新值替换选中的像素
modified_image = tf.clip_by_value(modified_image * 255.0, 0.0, 255.0) # 再次标准化到0-255范围内
return modified_image
# 保存图片
def save_image(modified_img, file_name):
with tf.io.gfile.GFile(file_name, "wb") as f:
f.write(tf.image.encode_jpeg(modified_img))
# 使用以上函数
img_path = "path/to/your/image.jpg"
image = read_and_decode_image(img_path)
modified_image = modify_pixels(image)
save_image(modified_image, "modified_" + img_path)
阅读全文
相关推荐
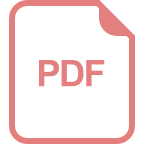
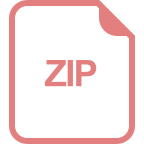

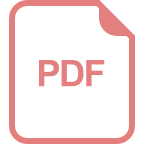
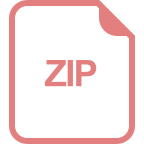
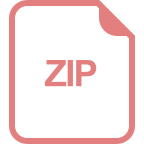
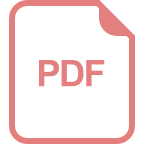
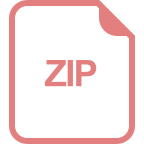
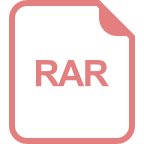
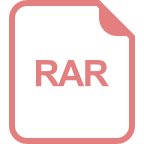
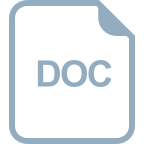



