给我生成一段用matlab修改图片像素为150X200的代码
时间: 2024-02-16 21:02:45 浏览: 111
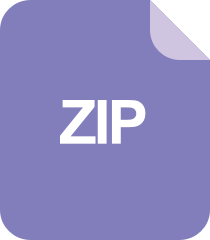
图像变换matlab代码
好的,请看下面的代码:
```matlab
% 读入图片
img = imread('input.jpg');
% 修改像素大小为150x200
img_resized = imresize(img, [150, 200]);
% 显示修改后的图片
imshow(img_resized);
% 保存修改后的图片
imwrite(img_resized, 'output.jpg');
```
这段代码会读入名为 `input.jpg` 的图片,将其像素大小修改为 150x200,并显示修改后的图片。最后,将修改后的图片保存为名为 `output.jpg` 的文件。
阅读全文
相关推荐
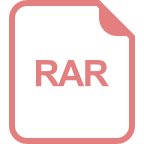
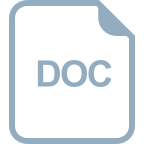












