使用 c++ dlib 最新版本写一段 人脸比对的代码 使用 ResNet
时间: 2024-01-12 16:05:37 浏览: 38
好的,以下是使用最新版本的dlib库,使用ResNet模型进行人脸比对的C++代码:
```c++
#include <iostream>
#include <dlib/dnn.h>
#include <dlib/data_io.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
using namespace std;
using namespace dlib;
// ResNet网络结构定义
// 使用dlib提供的ResNet网络结构,输入为150x150的RGB图像
using net_type = dlib::loss_metric<dlib::fc_no_bias<128, dlib::avg_pool_everything<
dlib::resnet<
dlib::num_filters<64>,
dlib::num_layers<121>,
dlib::net_type::relu,
dlib::input<dlib::matrix<dlib::rgb_pixel>>
>>>>;
int main()
{
try
{
// 加载已经训练好的ResNet模型
net_type net;
dlib::deserialize("dlib_face_recognition_resnet_model_v1.dat") >> net;
// 读取需要比对的两张图像
matrix<rgb_pixel> img1, img2;
load_image(img1, "img1.jpg");
load_image(img2, "img2.jpg");
// 对图像进行人脸检测和特征提取
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor sp;
dlib::deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
matrix<rgb_pixel> face1 = dlib::tile_images(dlib::extract_image_chips(dlib::get_face_chip_details(sp(img1, dlib::rectangle(detector(img1)[0]))), img1));
matrix<rgb_pixel> face2 = dlib::tile_images(dlib::extract_image_chips(dlib::get_face_chip_details(sp(img2, dlib::rectangle(detector(img2)[0]))), img2));
// 将特征提取的结果输入到ResNet网络中,得到人脸的128维特征向量
auto face1_embedding = net(face1);
auto face2_embedding = net(face2);
// 计算两个人脸特征向量的欧式距离,作为人脸相似度的度量
double distance = length(face1_embedding - face2_embedding);
cout << "The distance between the faces is: " << distance << endl;
}
catch (exception& e)
{
cout << e.what() << endl;
}
return 0;
}
```
这段代码使用dlib库中提供的ResNet网络结构进行人脸比对,输入为150x150的RGB图像,输出为128维的人脸特征向量。具体实现过程如下:
1. 加载已经训练好的ResNet模型,这里使用的是dlib库提供的已经训练好的ResNet模型。
2. 使用dlib库中的人脸检测器对需要比对的两张图像进行人脸检测,然后使用dlib库中的人脸特征提取器提取人脸区域,并将人脸区域resize为150x150的RGB图像。
3. 将特征提取的结果输入到ResNet网络中,得到人脸的128维特征向量。
4. 计算两个人脸特征向量的欧式距离,作为人脸相似度的度量。
注意:上面的代码中使用到了dlib库中的模型文件,需要先从dlib官网下载相应的模型文件。同时,为了提高比对的准确性,可以使用更加精细的人脸检测器和人脸特征提取器,这里只是提供一个简单的示例代码。
相关推荐
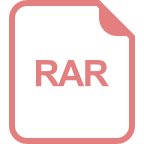










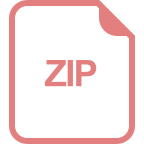
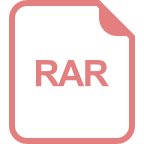
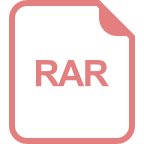