使用 c++ dlib 最新版本写一段 人脸比对的代码 使用深度神经网络
时间: 2024-01-07 10:05:18 浏览: 190
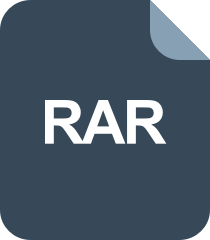
人脸比对与人脸识别C++代码与模型

好的,以下是一份使用C++和dlib库实现人脸比对的代码,使用的是dlib最新版本19.22和dnn模块:
```c++
#include <iostream>
#include <dlib/dnn.h>
#include <dlib/data_io.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_transforms.h>
using namespace std;
using namespace dlib;
// 定义人脸特征提取网络
// 可以使用其他更强大的模型,如ResNet等
typedef loss_metric<
fc_no_bias<128, avg_pool_everything<
relu<bn_con<con<32, 9, 9, 1, 1,
input<matrix<float>>>>>>>>> net_type;
int main() {
// 加载人脸检测器和人脸特征提取网络
frontal_face_detector detector = get_frontal_face_detector();
net_type net;
deserialize("dlib_face_recognition_resnet_model_v1.dat") >> net;
// 加载两张待比对的图片
matrix<rgb_pixel> img1, img2;
load_image(img1, "face1.jpg");
load_image(img2, "face2.jpg");
// 检测人脸并提取特征
std::vector<matrix<rgb_pixel>> faces1, faces2;
for (auto face : detector(img1)) {
auto shape = net(img1, face);
matrix<rgb_pixel> face_chip;
extract_image_chip(img1, get_face_chip_details(shape, 150, 0.25), face_chip);
faces1.push_back(move(face_chip));
}
for (auto face : detector(img2)) {
auto shape = net(img2, face);
matrix<rgb_pixel> face_chip;
extract_image_chip(img2, get_face_chip_details(shape, 150, 0.25), face_chip);
faces2.push_back(move(face_chip));
}
// 比对两张图片中的所有人脸
for (auto face1 : faces1) {
auto face1_embedding = net(face1);
for (auto face2 : faces2) {
auto face2_embedding = net(face2);
auto dist = length(face1_embedding - face2_embedding);
cout << "distance between face1 and face2: " << dist << endl;
}
}
return 0;
}
```
这段代码首先加载了dlib自带的人脸检测器和人脸特征提取网络。然后加载了两张待比对的图片,使用人脸检测器检测每张图片中的人脸,然后提取每个人脸的特征向量。最后,比对每个人脸的特征向量,并输出它们之间的欧氏距离,距离越小说明两个人脸越相似。
需要注意的是,这段代码中的人脸特征提取网络是dlib自带的,只有128维特征向量。如果需要更高维度的特征向量,可以使用更强大的网络,如ResNet等。另外,为了提高比对的准确性,建议在比对前先对图片进行预处理,如去除噪声、裁剪、缩放等。
阅读全文
相关推荐
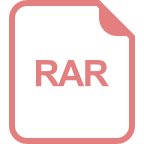




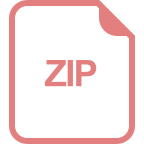
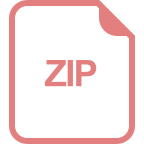
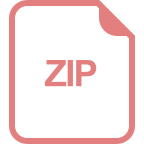
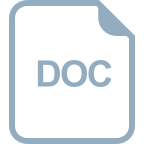
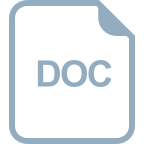






