写一段根据imu传感器读数来控制gazebo中立方体运动的程序的C++代码
时间: 2023-03-20 13:01:18 浏览: 199
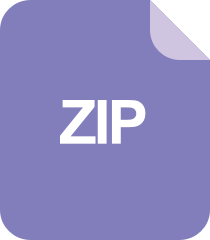
基于C++实现立方数码(AI实验)
以下是根据IMU传感器读数来控制Gazebo中立方体运动的C代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include <gazebo/gazebo.hh>
#include <gazebo/physics/physics.hh>
#include <gazebo/common/common.hh>
namespace gazebo
{
class ImuPlugin : public ModelPlugin
{
private:
physics::ModelPtr model;
physics::LinkPtr link;
event::ConnectionPtr updateConnection;
math::Vector3 angularVelocity;
public:
void Load(physics::ModelPtr _parent, sdf::ElementPtr /*_sdf*/)
{
// Save pointers to the model and link
this->model = _parent;
this->link = model->GetLink("link_name");
// Connect the update function to the simulation
this->updateConnection = event::Events::ConnectWorldUpdateBegin(boost::bind(&ImuPlugin::OnUpdate, this, _1));
// Set initial angular velocity
this->angularVelocity = math::Vector3(0, 0, 0);
}
void OnUpdate(const common::UpdateInfo &_info)
{
// Read IMU sensor data
math::Quaternion orientation = this->link->GetWorldPose().rot;
math::Vector3 angularVelocity = this->link->GetRelativeAngularVel();
// Control the cube's movement based on the IMU data
double roll, pitch, yaw;
orientation.GetAsEuler(roll, pitch, yaw);
double x_vel = -sin(yaw) * angularVelocity.y;
double y_vel = sin(roll) * cos(yaw) * angularVelocity.x + sin(pitch) * sin(yaw) * angularVelocity.y;
double z_vel = -cos(roll) * cos(yaw) * angularVelocity.x - sin(pitch) * cos(yaw) * angularVelocity.y;
// Apply the velocities to the cube's linear and angular velocity
this->link->AddRelativeForce(math::Vector3(x_vel, y_vel, z_vel));
this->link->AddRelativeTorque(math::Vector3(0, 0, 0.1));
}
};
GZ_REGISTER_MODEL_PLUGIN(ImuPlugin)
}
```
在这个示例中,我们创建了一个名为ImuPlugin的模型插件。在插件的Load()函数中,我们保存了模型和链接的指针,并将插件的更新函数与模拟连接。在更新函数OnUpdate()中,我们读取了IMU传感器数据,计算出立方体的线速度和角速度,并应用这些速度来控制立方体的运动。最后,我们在插件的最后注册了该插件。
请注意,此代码仅供参考,并不保证可以直接运行。为使该程序在您的机器上正常运行,您可能需要根据您的实际情况进行修改,并正确地链接Gazebo和相关库。
阅读全文
相关推荐


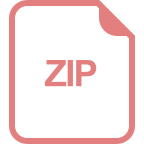
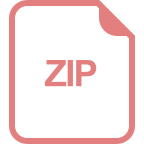
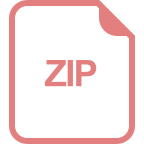
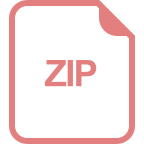
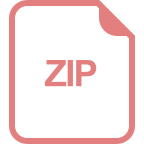
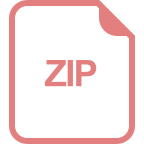
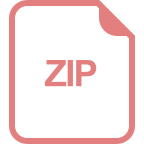
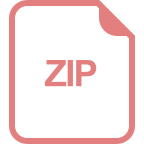
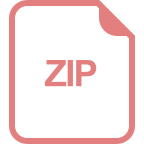
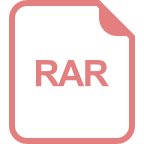
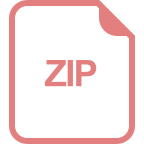
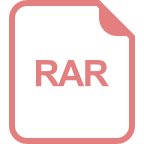
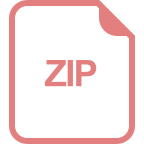