测验题目:错题修改 #include<stdio.h> void Swap(int *x,int *y); int main(void){ float a,b; scanf("%d,%d",&a,&b);/*输入a和b的值*/ printf("Before swap:a=%d,b=%d\n",a,b);/*打印交换前a,b的值*/ Swap(a,b); /*调用函数Swap实现a值与b值得交换*/ printf("After swap:a=%d,b=%d\n",a,b);/*打印交换后的a,b*/ } /*函数功能:交换两个整型数的值*/ void Swap(int *x,int *y); { int temp; temp = *x; *x = *y; *y = temp; }
时间: 2023-08-07 08:08:28 浏览: 79
这段代码中有一些错误,请看下面的修改后的代码:
```
#include <stdio.h>
void Swap(float *x, float *y);
int main(void) {
float a, b;
scanf("%f,%f", &a, &b); /*输入a和b的值*/
printf("Before swap: a=%f, b=%f\n", a, b); /*打印交换前a,b的值*/
Swap(&a, &b); /*调用函数Swap实现a值与b值得交换*/
printf("After swap: a=%f, b=%f\n", a, b); /*打印交换后的a,b*/
return 0;
}
/*函数功能:交换两个浮点型数的值*/
void Swap(float *x, float *y) {
float temp;
temp = *x;
*x = *y;
*y = temp;
}
```
修改之后的代码做了以下几个修改:
1. `%d` 应该改成 `%f`,因为 `a` 和 `b` 声明为了 `float` 类型;
2. 在函数声明和定义中,形参类型应该改成 `float *`,因为需要传递浮点型变量的地址;
3. 在函数定义中,去掉了多余的 `;` 符号;
4. 在 `main()` 函数中添加了 `return 0;` 语句,表示程序正常结束。
这样修改之后,代码就可以正确地编译、运行了。
相关问题
本题将5个字符串从小到大排序后输出(用指针数组实现)。 函数接口定义: void fsort(char *color[ ], int n); 其中 color为指针数组首地址,n是字符串个数。 裁判测试程序样例: #include <stdio.h> #include <string.h> void fsort(char *color[ ], int n); int main(void ) { int i; char *pcolor[ ] = {"red", "blue", "yellow", "green", "black"}; void fsort(char *color[ ], int n); fsort(pcolor, 5); /* 调用函数 */ for(i = 0; i < 5; i++) printf("%s ", pcolor[i]); printf("\n"); return 0; } /* 请在这里填写答案 */
题目要求用指针数组实现将5个字符串从小到大排序后输出。具体实现需要定义一个函数,函数的接口定义为:void fsort(char *color[ ], int n),其中color为字符串指针数组首地址,n是字符串个数。
下面是具体的裁判测试程序样例:
#include<stdio.h> int main(void) { int *p; *p=5; printf("%d",*p) }这串代码有什么问题
这段代码有指针未初始化的问题,指针变量 `p` 没有被赋值任何地址,因此在赋值 `*p=5` 时,程序会试图向未知的内存地址写入数据,这可能导致程序崩溃或者其他不可预知的错误。应该先为指针变量分配空间或者将其指向已经存在的变量地址,例如:
```c
#include <stdio.h>
int main(void) {
int a = 5;
int *p = &a; // p 指向变量 a 的地址
printf("%d", *p); // 输出 p 所指向的变量的值,即 a 的值 5
return 0;
}
```
阅读全文
相关推荐
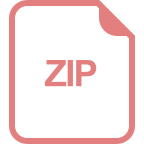
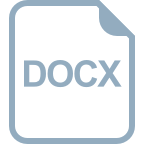
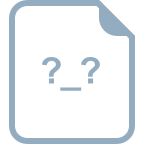














