请编写函数,求二叉树的结点个数。 函数原型 int BinTreeNumNode(const TNODE *root); 说明:root 为二叉树的根指针,函数值为二叉树的结点数。 在“BinTree.h”中声明函数,在“BinTree.c”中实现函数。 BinTree.h ...... int BinTreeNumNode(const TNODE *root); ...... BinTree.c ...... /* 你提交的代码将被嵌在这里 */ 打开“main.c”,修改主函数对以上函数进行测试。 main.c #include <stdio.h> #include "BinTree.h" int main() { TNODE *r; BinTreeCreate(&r); BinTreeInput(&r); printf("%d\n", BinTreeNumNode(r)); BinTreeDestroy(&r); return 0; } 题图.jpg 输入样例 EIBJ##H###DF#A##G#C## 输出样例 10
时间: 2024-02-07 13:02:35 浏览: 63
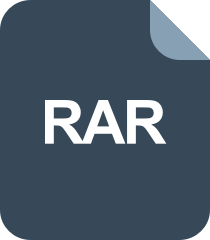
erchashu.rar_erchashu_统计 二叉树 结点个数

以下是实现二叉树结点个数的代码:
BinTree.h
```
#ifndef BINTREE_H
#define BINTREE_H
typedef struct tnode {
char data;
struct tnode *left;
struct tnode *right;
} TNODE;
void BinTreeCreate(TNODE **root);
void BinTreeInput(TNODE **root);
void BinTreeDestroy(TNODE **root);
int BinTreeNumNode(const TNODE *root);
#endif
```
BinTree.c
```
#include <stdlib.h>
#include "BinTree.h"
void BinTreeCreate(TNODE **root) {
*root = NULL;
}
void BinTreeInput(TNODE **root) {
char ch;
scanf("%c", &ch);
if (ch == '#') {
*root = NULL;
} else {
*root = (TNODE *) malloc(sizeof(TNODE));
(*root)->data = ch;
BinTreeInput(&((*root)->left));
BinTreeInput(&((*root)->right));
}
}
void BinTreeDestroy(TNODE **root) {
if (*root == NULL) {
return;
}
BinTreeDestroy(&((*root)->left));
BinTreeDestroy(&((*root)->right));
free(*root);
*root = NULL;
}
int BinTreeNumNode(const TNODE *root) {
if (root == NULL) {
return 0;
} else {
return 1 + BinTreeNumNode(root->left) + BinTreeNumNode(root->right);
}
}
```
main.c
```
#include <stdio.h>
#include "BinTree.h"
int main() {
TNODE *r;
BinTreeCreate(&r);
BinTreeInput(&r);
printf("%d\n", BinTreeNumNode(r));
BinTreeDestroy(&r);
return 0;
}
```
阅读全文
相关推荐
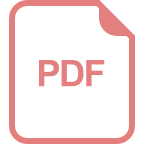
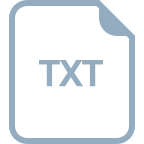















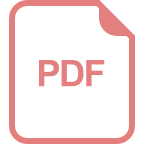